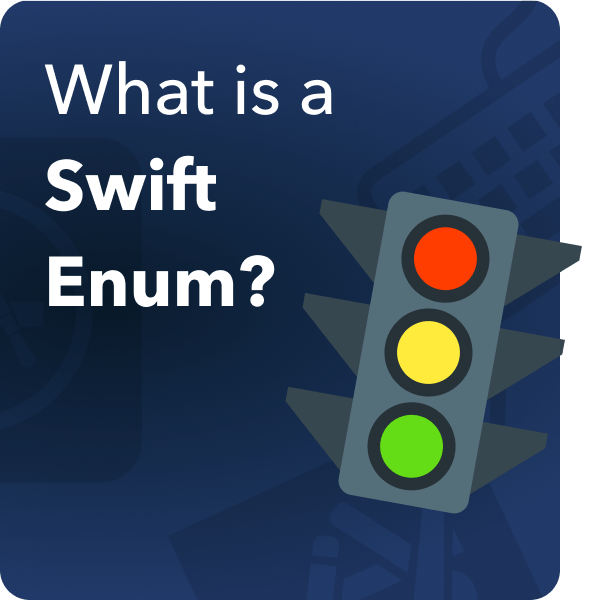
You are probably familiar what a Data Type is, you might even be familiar what a Class and a Structure is, but what you seldom see is an Enum.
So, what are are Enums and how does it work? This article will be discussing all about Enumeration in swift.
What is a Swift Enum?
According to the Swift documentation enumeration is defined as “a common type for a group of related values and enables you to work with those values in a type-safe way within your code”.
Think of it as a type of variable that is specifically used switch/conditionals.
Switch Usage
To better understand let us demonstrate with an example. Say we make an enum for directions:
enum MoveDirection { case forward case back case left case right }
Now that we have established an enum we can use it like this:
var movement = MoveDirection.left; switch movement { case .forward: print("you moved forward") case .back: print("you moved backwards") case .left: print("you moved to the left") case .right: print("you moved to the right") } //this will print out “you moved to the left”
Given this example we can then improve it and maybe use it in a game. How about we put the switch statement inside a function called doMove that accepts MoveDirection as a parameter:
func doMove(_ movement:MoveDirection){ switch movement { case .forward: print("you moved forward") case .back: print("you moved backwards") case .left: print("you moved to the left") case .right: print("you moved to the right") } } var action = MoveDirection.left; doMove(action) //this will print out “you moved to the left”
We can even just simply assign action again with just the direct use case like so:
action = .forward toMove(action) //this will print out “you moved forward”
Or you can even just directly input the case to the function like this:
toMove(.right) //this will print out “you moved to the right”
As you can see enumeration is highly versatile and is great for using in switch cases given the example.
Enums with Values
User-defined
We have demonstrated how enum was used for switch or conditions, but can it hold a value? Yes it can! You call predefined values as rawValue. Let’s try and demonstrate by improving our old example and adding a value to it.
It should also be noted that you need to assign a Data Type to your enum in order to set a value to it.
enum MoveDirection : String { case forward = "you moved forward" case back = "you moved backwards" case left = "you moved to the left" case right = "you moved to the right" }
Since we have defined a value to the enum we can simply use .rawValue to get the value of the item:
var action = MoveDirection.left; print(action.rawValue) // this will print out "you moved to the left"
But doesn’t typing rawValue kind of weird? Well there’s another way, you can change by defining a function inside the enum:
enum MoveDirection : String { case forward = "you moved forward" case back = "you moved backwards" case left = "you moved to the left" case right = "you moved to the right" func printDirection() -> String { return self.rawValue } } var action = MoveDirection.right; print(action.printDirection())// this will print out "you moved to the right"
Implicit Assignment
Now we know we can define each value of an enum individually, but what if we just want a series of numbers to determine a score? Wouldn’t that be a pain to type individually? Not to mention messy. We can use Implicit assignment for that!. Here is a sample:
enum GolfScores : Int { case HoleInOne = 1, Birdie, Boogie, Par4, Par5, Par6 func getScore() -> Int { return self.rawValue } } var shot = GolfScores.Birdie print(shot.getScore()) // this will print out 2
As you can see we didn’t need to assign the values for each case individually as long as it is in a series, very handy!
Associated Values
We learned that enums have predefined values and you can access them by using .rawValue, but what if you want to assign the values yourself? Here is when associated values come into play. The usage and set-up is a little weird so it’s not for the faint of heart.
In order to make an associated value for enum just set the Data Types to receive for each case like this:
enum BankDeposit { case inValue(Int, Int, Int) //hundreds,tens,ones case inWords(String) //words }
Notice that the enum itself doesn’t have a type unlike the user-defined value, this is because we can receive any Data Type for each case or even multiple values.
Now in-order to make use of our enum we need to make a specialized switch for it. Let’s spice it up and put it in a function named makeDeposit so we can easily reuse it.
func makeDeposit(_ person:BankDeposit){ switch person{ case .inValue(let hundred, let tens, let ones): print("deposited: \((hundred*100)+(tens*10)+(ones*1))") case .inWords(let words): print("deposited: \(words)") } }
You might have noticed that in each of our cases we needed to assign a constant to capture the data, you should also notice that we can manipulate the data like in the inValue example where we needed to compute for the total value based on hundred, tens, and ones input.
We are now ready to use our enum and switch, lets make two variables so we can demonstrate both cases:
var person1 = BankDeposit.inValue(1,2,5) makeDeposit(person1) //prints deposited: 125 var person2 = BankDeposit.inWords("One Hundred Twenty Five") makeDeposit(person2) //prints deposited: One Hundred Twenty Five
And viola! you have now learned how to make enums with associated values.
Conclusion
That’s about it for enums in Swift really, there’s also a functionality called “Recursive Enumerations” according to the Swift documentation but I think that would be too much to handle, let’s let the pros and mathematicians handle that one. However, if you are really curious here is the link to the documentation. Happy coding! 🙂
Further Reading
- The Complete Swift Tutorial for Beginners: Learn Swift programming with my Swift guide designed with the beginner in mind.
- Cocoapods Tutorial: Learn how to install and use Cocoapods in your Xcode project! Take advantage of third party Swift libraries and GitHub repositories easily.
- Swift Timer: Learn how to easily schedule and run a Swift timer and how to configure the Swift timer for various use cases and scenarios.
- 7 Day App Action Plan: Plan out your app and finally get started turning your app idea into reality.