Overview
This article explains how to create menus in SwiftUI using the Menu
view. It covers adding actions to menu items, customizing the appearance of menu buttons, and integrating menus into different parts of your SwiftUI layout.
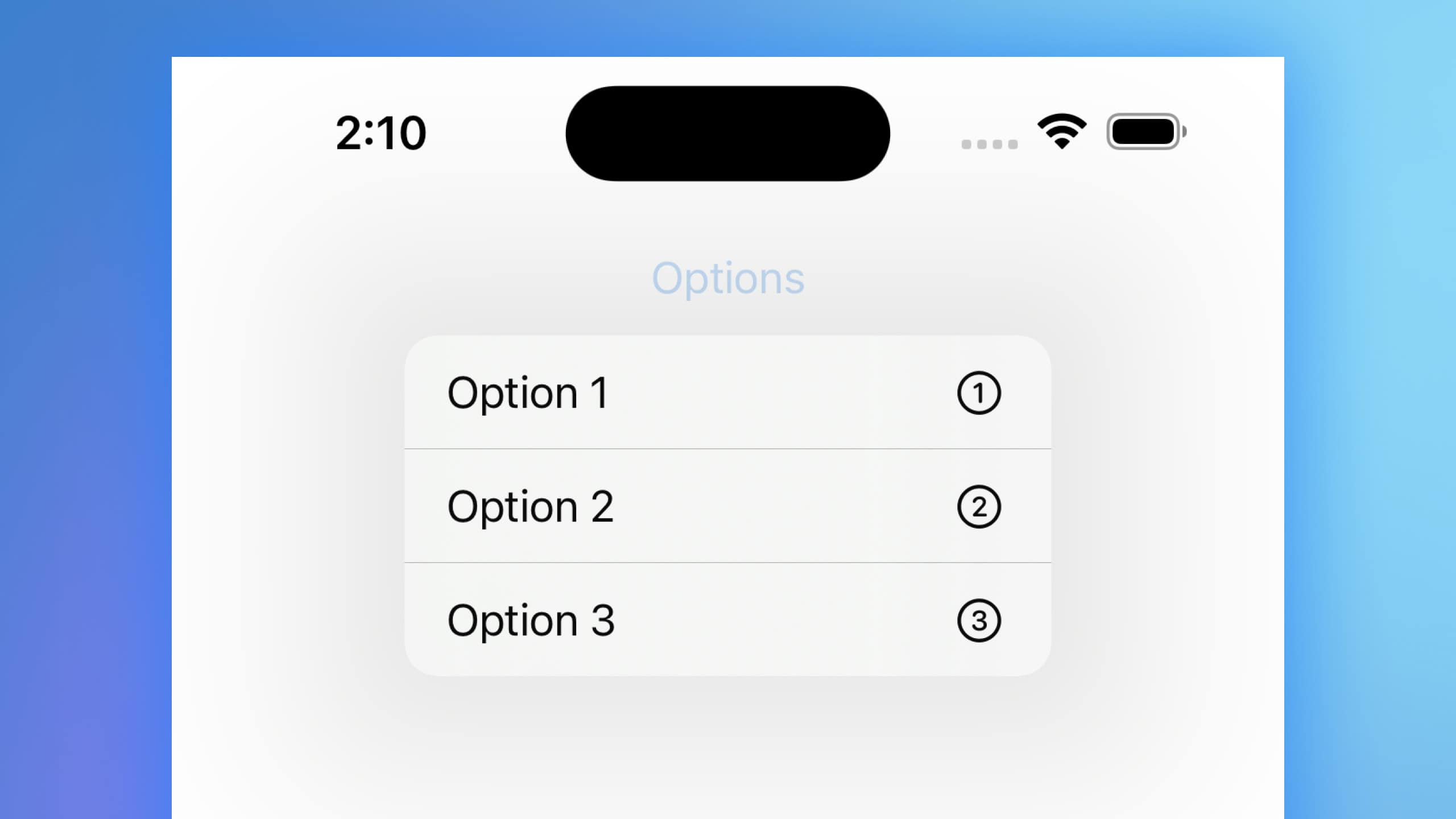
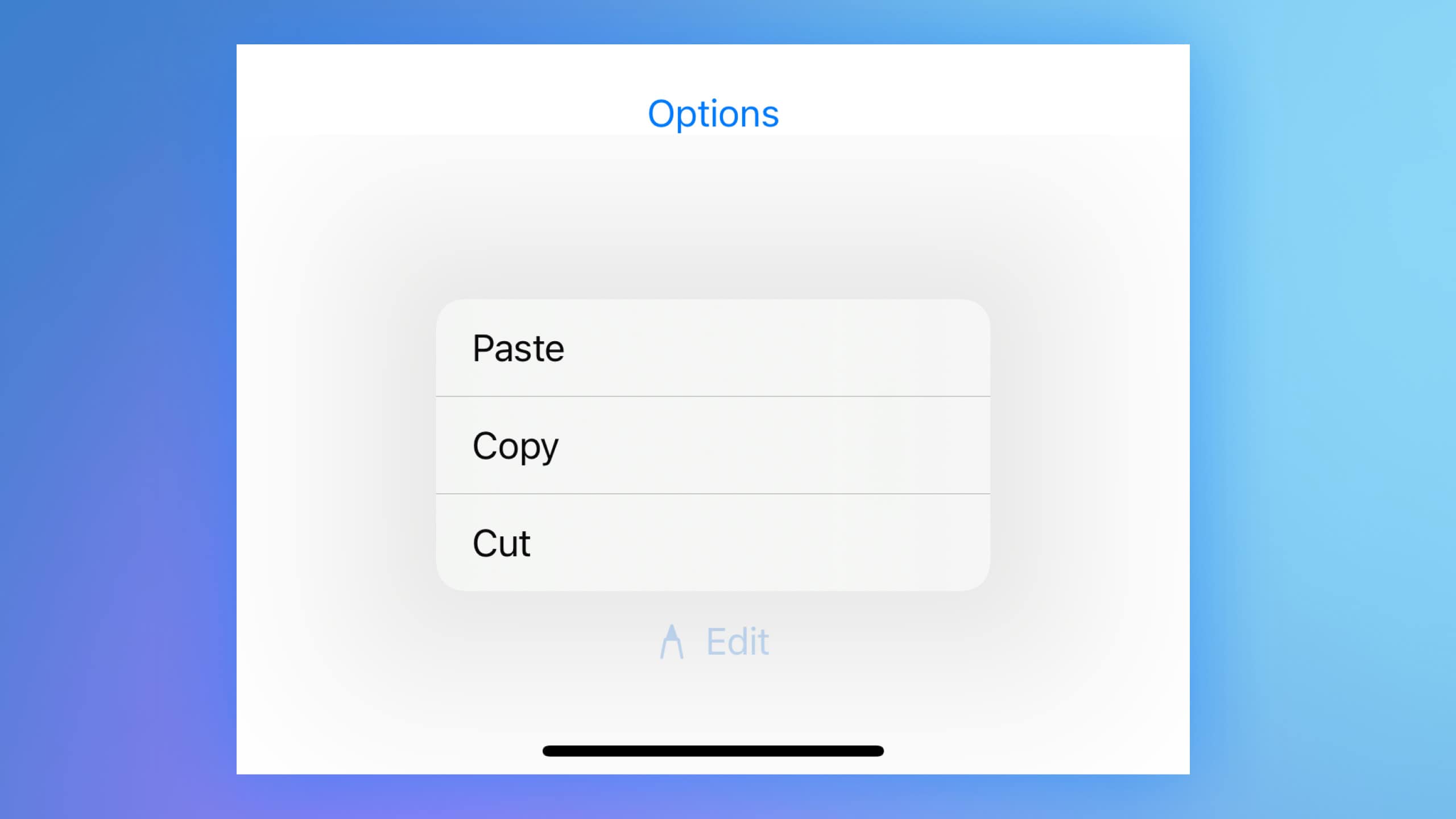
Code Snippet
import SwiftUI
struct MenuView: View {
var body: some View {
VStack {
Menu("Options") {
Button {
print("Option 1 selected")
} label: {
Label("Option 1", systemImage: "1.circle")
}
Button {
print("Option 2 selected")
} label: {
Label("Option 2", systemImage: "2.circle")
}
Button {
print("Option 3 selected")
} label: {
Label("Option 3", systemImage: "3.circle")
}
}
.menuStyle(.borderlessButton)
.padding()
Spacer()
Menu {
Button("Cut", action: cut)
Button("Copy", action: copy)
Button("Paste", action: paste)
} label: {
Label("Edit", systemImage: "pencil.tip")
}
.padding()
}
}
func cut() {
print("Cut action")
}
func copy() {
print("Copy action")
}
func paste() {
print("Paste action")
}
}
Code Explanation
Menu("Options")
: Creates a menu with the title “Options”. This menu contains multiple buttons as its items.Button { print("Option 1 selected") } label: { Label("Option 1", systemImage: "1.circle") }
: Creates a menu item labeled “Option 1” with an image of the number 1 inside a circle. Theaction
closure prints a message to the console when the item is selected.- Similarly, other buttons are created for “Option 2” and “Option 3” with their respective actions and icons.
.menuStyle(.borderlessButton)
: Applies a borderless style to the menu, making it visually consistent with other controls and removing the default border..padding()
: Adds padding around the menu for better layout spacing.Menu { } label: { }
: This syntax is used to create a menu with a custom label.- The
Menu
initializer here is provided with a trailing closure for thelabel
, which defines how the menu button looks. Label("Edit", systemImage: "pencil.tip")
: Sets the label of the menu button to “Edit” with a pencil tip icon.- Inside the
Menu
block, three buttons are defined:Button("Cut", action: cut)
: Creates a button labeled “Cut” which calls thecut
function when selected.Button("Copy", action: copy)
: Creates a button labeled “Copy” which calls thecopy
function when selected.Button("Paste", action: paste)
: Creates a button labeled “Paste” which calls thepaste
function when selected.
- The
func cut()
,func copy()
,func paste()
: These functions define the actions for the respective menu items. Each function prints a message to the console when invoked, indicating which action was performed.
Menus in SwiftUI provide a powerful way to offer multiple actions to users within a single button. They are highly customizable, both in terms of appearance and functionality, making them suitable for a variety of applications. Whether you need a simple options menu or a more complex set of actions, SwiftUI’s Menu
view offers a flexible and easy-to-use solution.