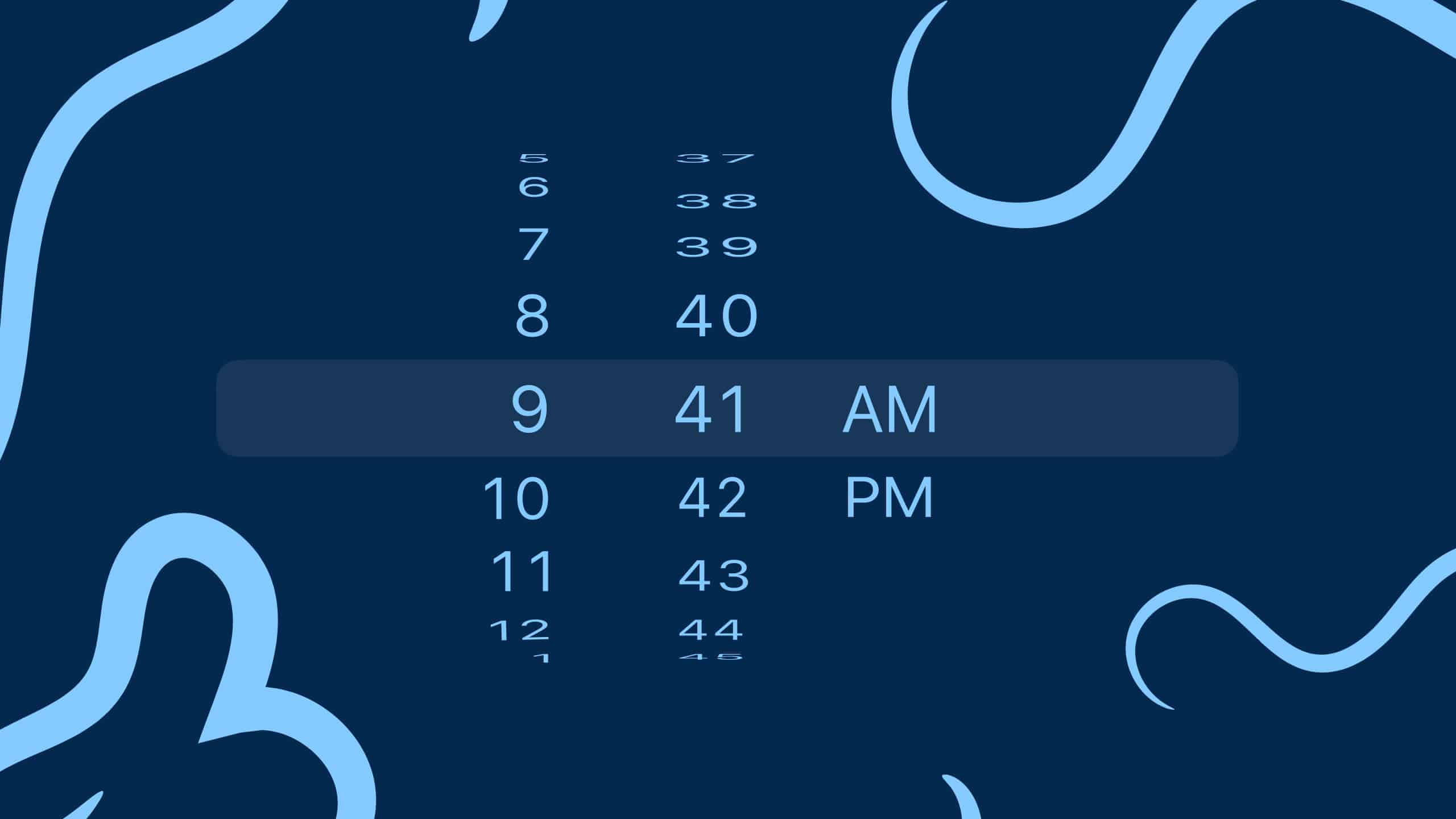
Written by Iñaki Narciso
Written for Swift 5.7 using Xcode 14.2
This tutorial aims to recreate the UIPickerView Example and Tutorial (Updated 2018) in SwiftUI using the Picker view.
A Picker is a control that allows selecting a single value from a set of values, similar to what a dropdown does for a webpage. This tutorial will explore how to use a Picker, add it to a SwiftUI view, and make a selection.
Prerequisites
To follow this tutorial, you’ll need to have a Mac with Xcode installed.
Don’t have a Mac computer? Check out your options using Windows.
We’ll also cover the use of Swift enums
to declare the data we’ll use for the Picker
.
If you’re new and haven’t used Swift enums
before, you may want to check the Swift enums four-part series first: Part 1, Part 2, Part 3, and Part 4.
Overview
Step 1: Creating the Xcode Project
Start by creating the Xcode project, and choosing App
from the iOS
template tab.
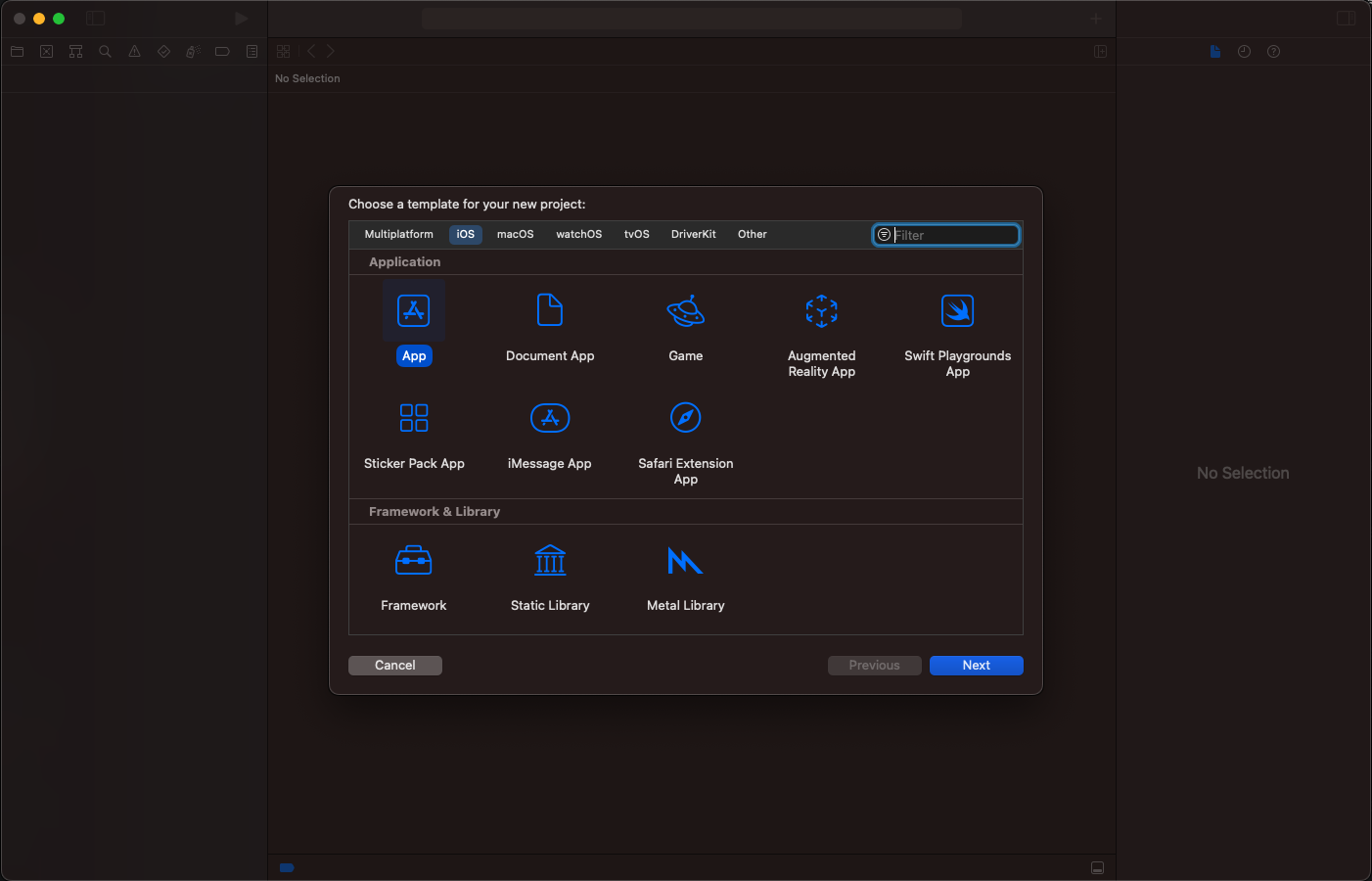
Configure the project by making sure the interface
selected is SwiftUI
.
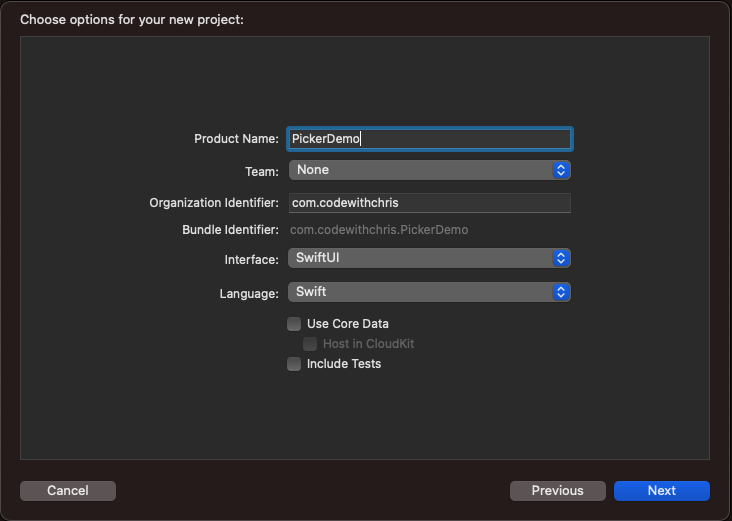
Click on Next
and choose a folder where you would save your project.
Step 2: Declaring the Data as Enums
To create a picker, we need:
- An enum data as the content of the picker
- A default value from the enum data, set as a selection binding
- A label
Let’s begin by creating the picker data first as we’ll use it as the basis for the default value. To make this tutorial beginner-friendly, we’ll put all code inside the ContentView.swift
.
The data we’ll use for this tutorial would be the names of the planets in our Solar system. By default, we’ll select Earth
as the default value of the picker, and allow selecting a new planet that would change the selected value of the picker.
In Xcode, open ContentView.swift
:
import SwiftUI struct ContentView: View { // Begin by declaring the data as enums enum Planet: String, CaseIterable, Identifiable { case mercury, venus, earth, mars, jupiter, saturn, uranus, neptune var id: Self { self } } var body: some View { ... } } struct ContentView_Previews: PreviewProvider { ... }
We’ll declare the default value of the picker by selecting an enum
value and using it as the default value of the Picker.
import SwiftUI struct ContentView: View { enum Planet: String, CaseIterable, Identifiable { case mercury, venus, earth, mars, jupiter, saturn, uranus, neptune var id: Self { self } } // We'll select Earth as the default value of the Picker @State private var selectedPlanet: Planet = .earth var body: some View { ... } } struct ContentView_Previews: PreviewProvider { ... }
Now that we have the data to show to the picker and a default value to set, let’s proceed with adding the Picker to the UI.
Step 3: Adding the Picker to the View
To add the Picker to the view, remove the code inside the body
and change it to:
struct ContentView: View { enum Planet: String, CaseIterable, Identifiable { case mercury, venus, earth, mars, jupiter, saturn, uranus, neptune var id: Self { self } } @State private var selectedPlanet: Planet = .earth var body: some View { List { Picker("Planet", selection: $selectedPlanet) { ForEach(Planet.allCases) { planet in Text(planet.rawValue.capitalized) } } } } } struct ContentView_Previews: PreviewProvider { ... }
We wrap the Picker
on a List
so the List
would show the Picker
at the top instead of showing it in the center like using a VStack
.
Picker with Default Value | Picker Showing Data For Selection |
---|---|
![]() | ![]() |
Selecting a new value from the Picker will assign a new value for selectedPlanet
. Initially, the selectedPlanet
binding will determine the value of the picker, but when the value changes due to a new selection, the Picker will drive the value back to the selectedPlanet
state property.
Step 4: Configuring the Picker using PickerStyle
The Picker, by default, would show an inline view with the selected value, and tapping it would show a popover menu for all possible options.
Selecting a value from the popover menu will change the selected value of the inline picker.
When pickerStyle
isn’t set to the Picker, the default value would be .automatic
.
Using PickerStyle
, we can specify how the Picker
should look like.
Inline Picker Style
struct ContentView: View { ... var body: some View { List { Picker("Planet", selection: $selectedPlanet) { ForEach(Planet.allCases) { planet in Text(planet.rawValue.capitalized) } } } .pickerStyle(.inline) } }
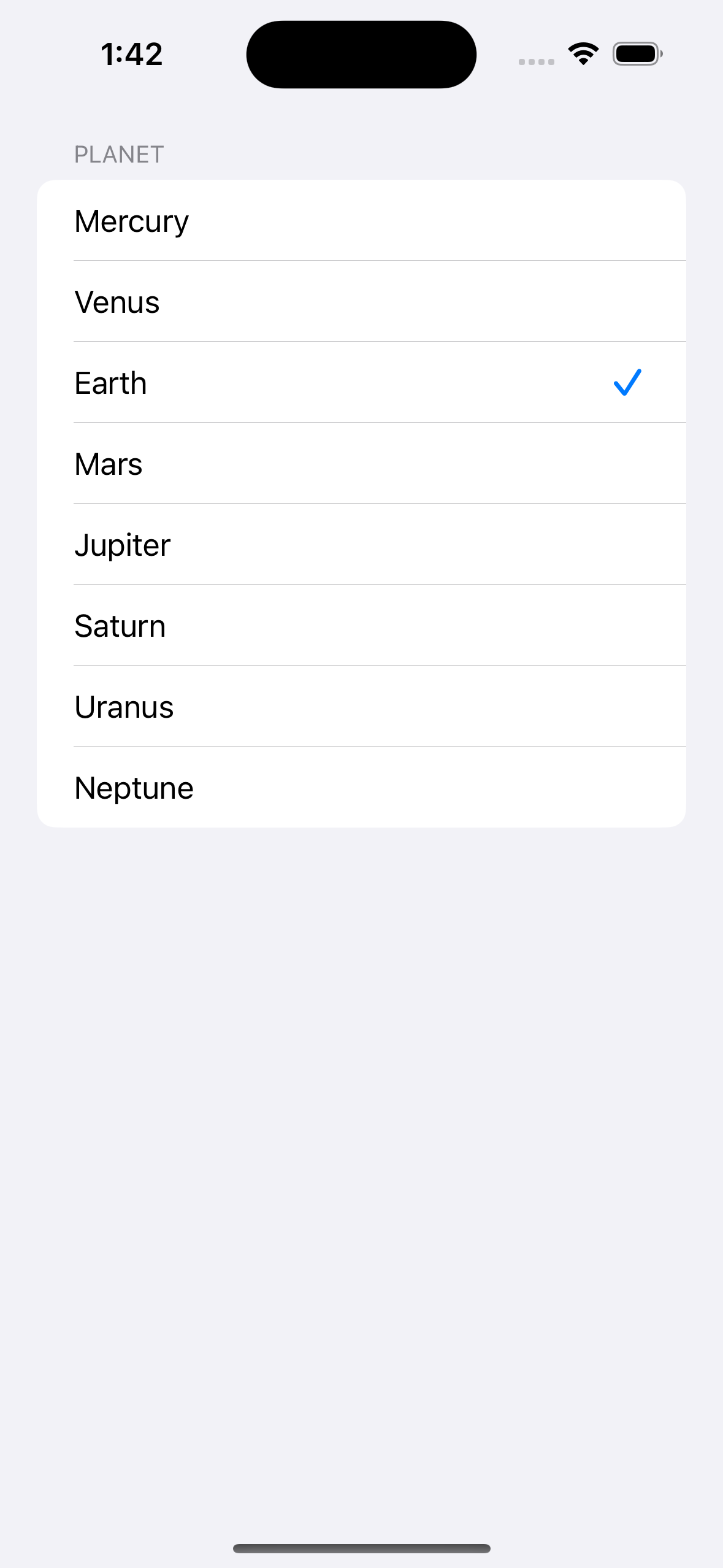
Wheel Picker Style
This is a style similar to the UIPickerView.
struct ContentView: View { ... var body: some View { List { Picker("Planet", selection: $selectedPlanet) { ForEach(Planet.allCases) { planet in Text(planet.rawValue.capitalized) } } } .pickerStyle(.wheel) } }
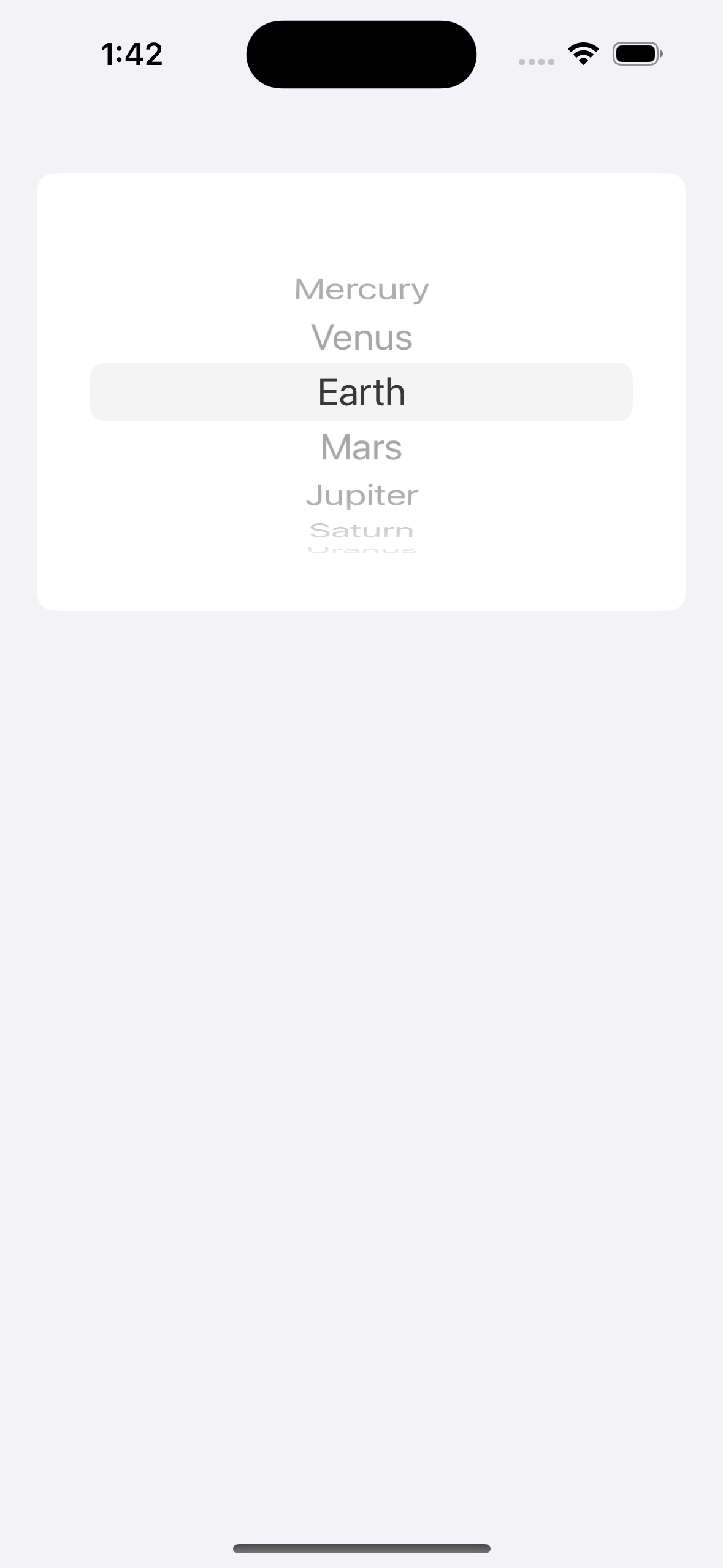
Navigation Link Picker Style
The navigation link picker style is ideal when you want to present a new screen for selection.
This picker style requires a NavigationStack
as the root view of the body
otherwise, it wouldn’t work.
struct ContentView: View { ... var body: some View { NavigationStack { List { Picker("Planet", selection: $selectedPlanet) { ForEach(Planet.allCases) { planet in Text(planet.rawValue.capitalized) } } } } .pickerStyle(.navigationLink) } }
Picker with Default Value | Picker Showing Data For Selection |
---|---|
![]() | ![]() |
Segmented Picker Style
A segmented picker style is like a Tab selection. This is not an ideal picker style when you have more than three options as mobile devices tend to have limited width especially in portrait orientation.
struct ContentView: View { ... var body: some View { List { Picker("Planet", selection: $selectedPlanet) { ForEach(Planet.allCases) { planet in Text(planet.rawValue.capitalized) } } } .pickerStyle(.segmented) } }
Showing three values | Showing all values |
---|---|
![]() | ![]() |
Notice that when I attempt to show all options, the label for each option becomes truncated and is no longer visually pleasing.
So only use this picker style when you have 3 options or fewer.
Conclusion
The Picker control follows the design standards Apple made for the Human Interface Guidelines for Input and Selection.
Creating a Picker is easier in SwiftUI. It involves less code and setup compared to doing it in UIKit. I hope that by following this tutorial, you get to understand how to use a Picker on your projects!
That’s all for now. Happy learning!