Overview
This article introduces the ShareLink
in SwiftUI, a convenient tool for enabling sharing capabilities within your app. ShareLink
allows users to share content such as text, URLs, images, and more through the standard iOS share sheet. This feature simplifies the process of integrating sharing functionality, making it seamless for users to share content from your app with others.
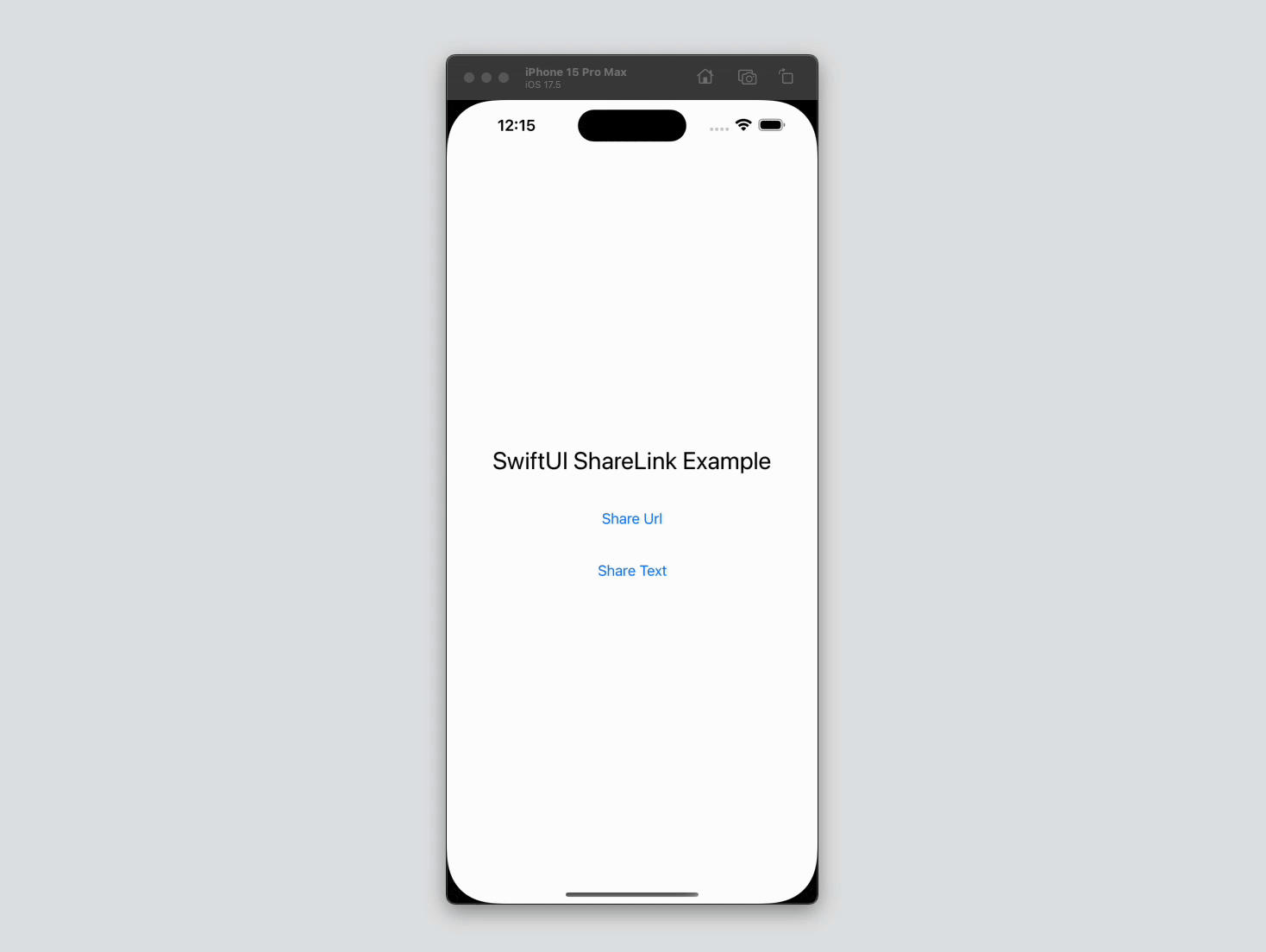
Code Snippet
import SwiftUI
struct ShareLinkExample: View {
let textToShare = "Check out this amazing content!"
let urlToShare = URL(string: "https://www.example.com")!
var body: some View {
VStack {
Text("SwiftUI ShareLink Example")
.font(.title)
.padding()
ShareLink(item: urlToShare) {
Text("Share URL")
}
.padding()
ShareLink(item: textToShare) {
Text("Share Text")
}
.padding()
}
}
}
Code Explanation
let textToShare = "Check out this amazing content!"
: Defines a constant string that will be shared.let urlToShare = URL(string: "https://www.example.com")!
: Defines a constant URL that will be shared. The URL is force unwrapped for simplicity, assuming it’s always valid.ShareLink(item: urlToShare) { ... }
: Creates a ShareLink for sharing the URL. Theitem
parameter is the content to be shared, and the trailing closure provides the view to be displayed for initiating the share action.Text("Share URL")
: The view displayed for sharing the URL. In this case, it’s a simple text label.ShareLink(item: textToShare) { ... }
: Creates a ShareLink for sharing the text. Theitem
parameter is the content to be shared, and the trailing closure provides the view to be displayed for initiating the share action.Text("Share Text")
: The view displayed for sharing the text. Again, it’s a simple text label.
Controlling What You Share
You can control what you share by customizing the item provided to ShareLink
. For example, you can share different types of content based on user interaction or app state.
Code Snippet
import SwiftUI
struct ConditionalShareLinkExample: View {
@State private var shareText = false
let textToShare = "Check out this amazing content!"
let urlToShare = URL(string: "https://www.example.com")!
var body: some View {
VStack {
Text("Conditional ShareLink Example")
.font(.title)
.padding()
Toggle("Share Text", isOn: $shareText)
.padding()
if shareText {
ShareLink(item: textToShare) {
Text("Share Text")
}
.padding()
} else {
ShareLink(item: urlToShare) {
Text("Share URL")
}
.padding()
}
}
}
}
Code Explanation
@State private var shareText = false
: Declares a state variable to track whether text should be shared.Toggle("Share Text", isOn: $shareText)
: A toggle switch that binds toshareText
, allowing the user to switch between sharing text and sharing a URL.if shareText { ... } else { ... }
: A conditional statement that determines which content to share based on the value ofshareText
.ShareLink(item: textToShare) { ... }
: Creates a ShareLink for sharing the text whenshareText
is true.ShareLink(item: urlToShare) { ... }
: Creates a ShareLink for sharing the URL whenshareText
is false.
Customizing the Share Sheet
To customize the content that appears in the share sheet, you can use ShareLink
with a custom data type. Here’s an example of sharing a custom message with additional metadata. For more detailed guidelines on customizing the Activity View (Share Sheet), refer to the Human Interface Guidelines for Activity Views.
Code Snippet
import SwiftUI
struct CustomData: Identifiable {
let id = UUID()
let title: String
let description: String
}
struct CustomShareLinkExample: View {
let customData = CustomData(title: "Amazing Content",
description: "This is an amazing piece of content you should check out!")
var body: some View {
VStack {
Text("Custom ShareLink Example")
.font(.title)
.padding()
ShareLink(item: customData.description) {
Text("Share Custom Data")
}
.padding()
}
}
}
Code Explanation
struct CustomData: Identifiable { ... }
: Defines a custom data type conforming to theIdentifiable
protocol.let customData = CustomData(title: "Amazing Content", description: "This is an amazing piece of content you should check out!")
: Creates an instance ofCustomData
with a title and description.ShareLink(item: customData.description) { ... }
: Creates a ShareLink for sharing the custom data’s description. Theitem
parameter is the content to be shared, and the trailing closure provides the view to be displayed for initiating the share action.Text("Share Custom Data")
: The view displayed for sharing the custom data. It’s a simple text label indicating the action.
The ShareLink
component in SwiftUI provides a straightforward way to incorporate sharing functionality into your app. By enabling users to share text, URLs, images, and other content through the standard iOS share sheet, ShareLink
enhances the user experience and makes it easier for users to distribute content from your app. Utilizing ShareLink
can significantly increase the reach and engagement of the content within your app, and with the ability to control and customize what is shared, you can provide a tailored and rich sharing experience.