Overview
In SwiftUI, Sheets are used to present modals that slide up from the bottom of the screen. They provide a way to present new content without pushing a new view onto the navigation stack. Sheets are commonly used for presenting additional information, forms, or any other content that temporarily takes over part of the screen. This article will walk you through creating and presenting a simple sheet using SwiftUI.
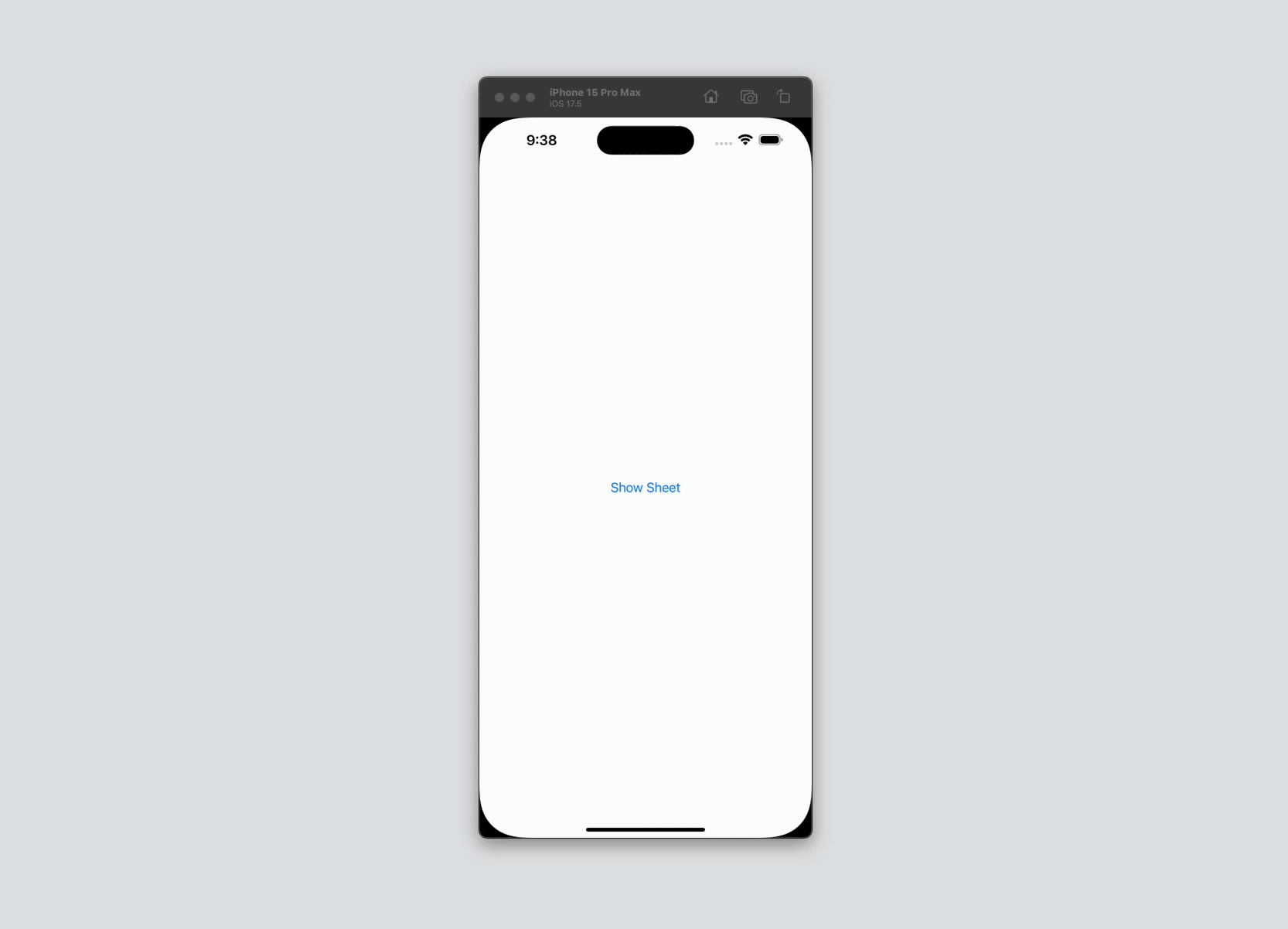
Code Snippet
import SwiftUI
struct ContentView: View {
@State private var showingSheet = false // State to control sheet presentation
var body: some View {
VStack {
Button("Show Sheet") {
showingSheet.toggle() // Toggle the state to show the sheet
}
}
.sheet(isPresented: $showingSheet, content: {
SheetView() // Content of the sheet
})
.padding()
}
}
struct SheetView: View {
@Environment(\.dismiss) var dismiss
var body: some View {
VStack {
Text("This is a sheet")
.font(.largeTitle)
.padding()
Button("Dismiss") {
dismiss()
}
}
}
}
Code Explanation
@State private var showingSheet = false
: This line declares a state variable that controls whether the sheet is presented. It is initialized tofalse
because we do not want the sheet to be visible initially.Button("Show Sheet") { showingSheet.toggle() }
: This button toggles theshowingSheet
state variable. When pressed, it changes the state fromfalse
totrue
, thereby presenting the sheet..sheet(isPresented: $showingSheet, content: { SheetView() })
: This modifier attaches a sheet to the view. It takes a binding toshowingSheet
, meaning the sheet will be presented whenshowingSheet
istrue
and dismissed when it isfalse
. The closure{ SheetView() }
defines the content of the sheet.@Environment(\.dismiss) var dismiss
: This line declares an environment variable to handle the dismissal of the sheet.Button("Dismiss") { dismiss() }
: This button calls thedismiss
function to close the sheet when pressed.
Sheets in SwiftUI provide a straightforward way to present modals. They are highly customizable and can contain any SwiftUI view, making them versatile for various use cases. Whether you need to present a simple message or a complex form, Sheets in SwiftUI offer a clean and efficient solution for your app’s user interface.