Overview
The SwiftUI Stepper is a user interface component that allows users to increment or decrement a value by tapping a plus or minus button. This article explores how to implement and customize a Stepper in SwiftUI, including setting its range and handling its value changes programmatically.
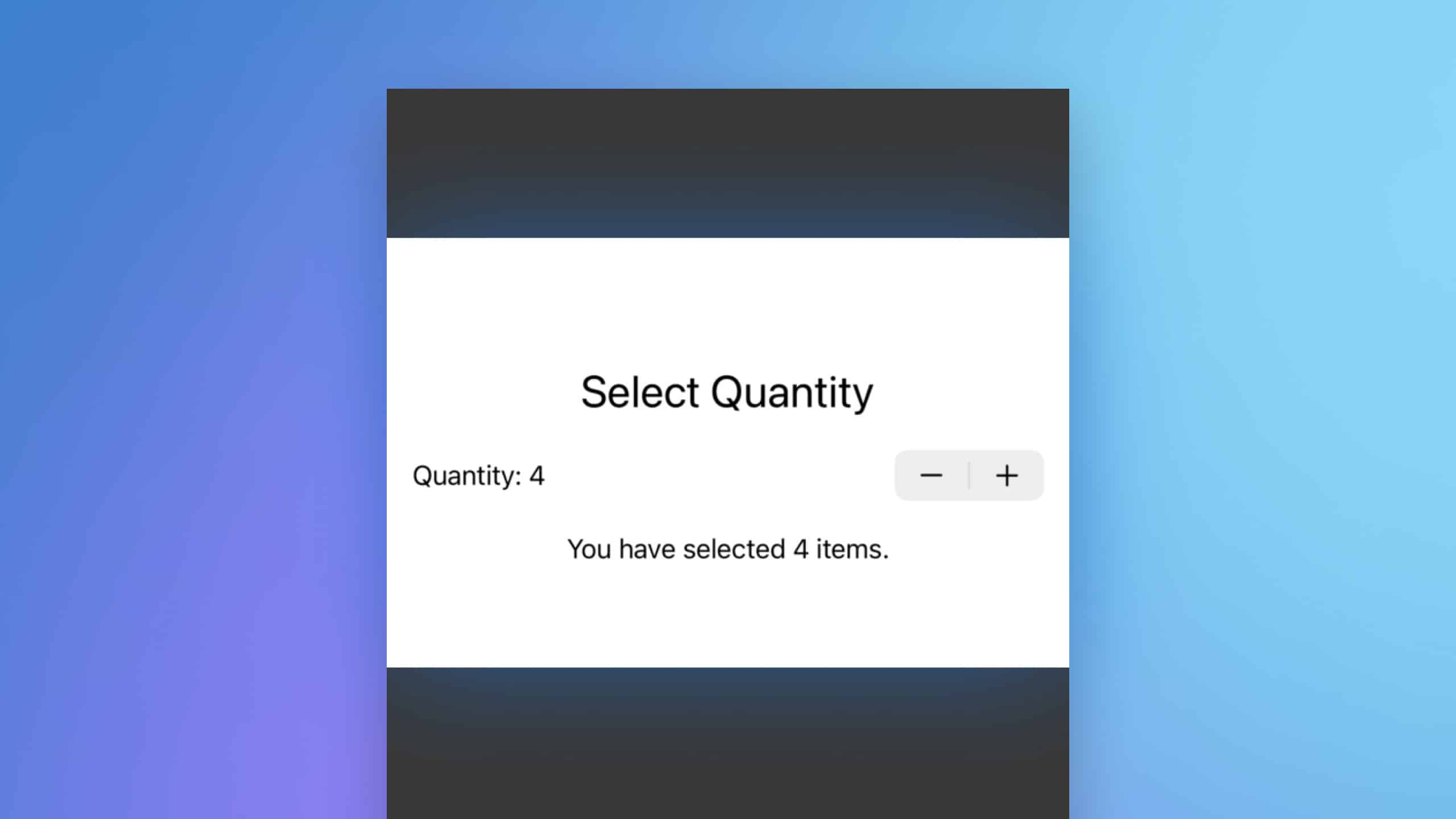
Code Snippet
import SwiftUI
struct StepperView: View {
@State private var quantity = 1
var body: some View {
VStack(spacing: 20) {
Text("Select Quantity")
.font(.title)
Stepper("Quantity: \(quantity)", value: $quantity, in: 1...10)
Text("You have selected \(quantity) items.")
}
.padding()
}
}
Code Explanation
@State private var quantity = 1
: This line initializes a state variablequantity
that will store the current value shown by the Stepper.Stepper("Quantity: \(quantity)", value: $quantity, in: 1...10)
: The Stepper is created with a text label that displays the current quantity. It binds to thequantity
variable and allows values from 1 to 10.- The view displays the current value of
quantity
alongside a label, updating in real-time as the Stepper is used.
This code example demonstrates how to use a Stepper to adjust the quantity of items, with the user being able to increase or decrease the number between 1 and 10. It showcases the binding of SwiftUI state variables to user interface elements for interactive and dynamic UI updates.
The SwiftUI Stepper is an essential component for creating interfaces where users need to adjust values within a defined range. It is versatile, easy to implement, and can be customized to fit the needs of various applications, making it a valuable tool for developers building user-centric apps.