Overview
Swipe actions in SwiftUI provide a direct way for users to interact with list items through gestures that perform actions like delete or edit. This feature enhances the user interface by allowing quick actions that are both intuitive and efficient. Here’s how you can fully implement swipe actions in your SwiftUI app.
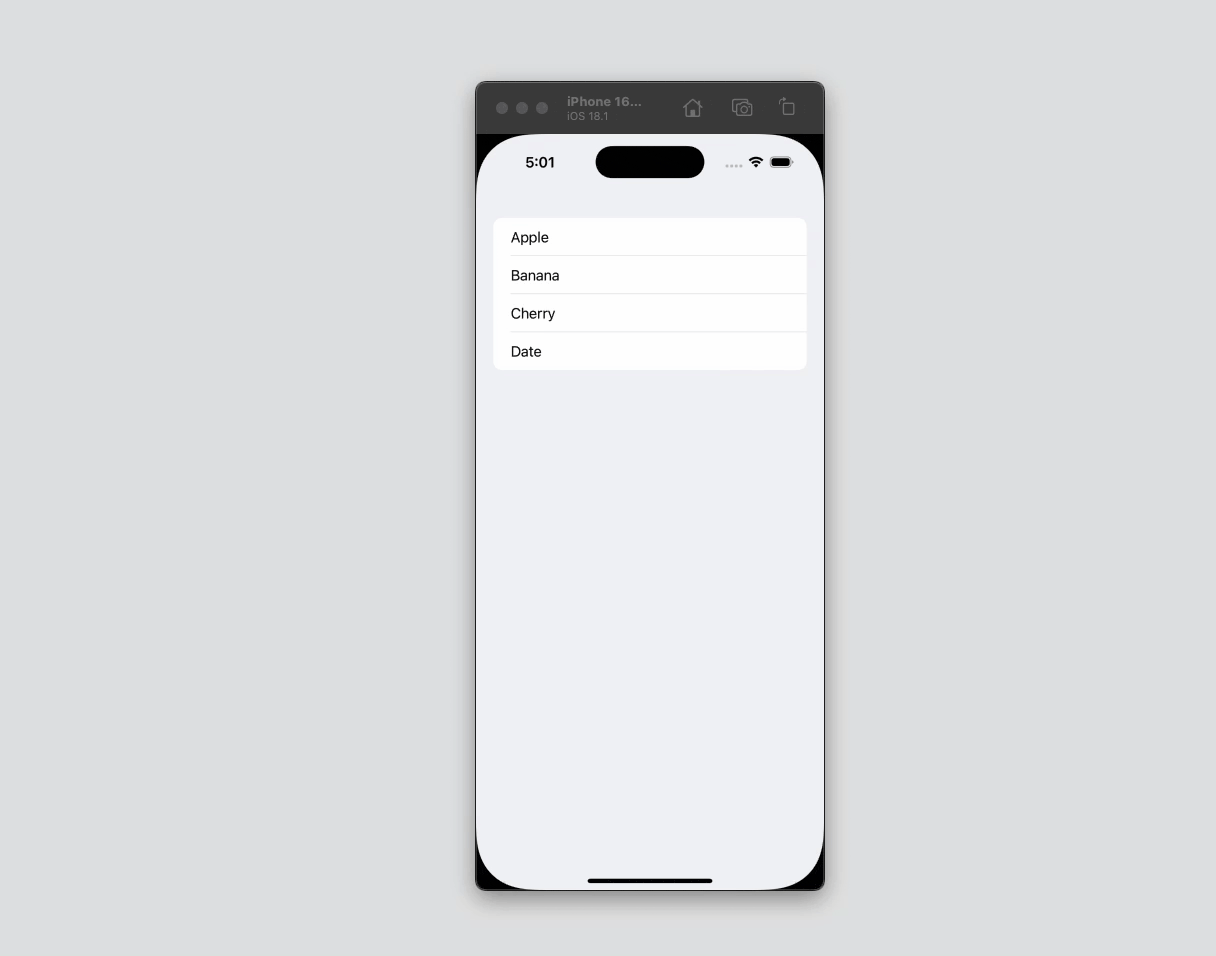
Creating the SwiftUI View
To set up swipe actions, you start with a List
in SwiftUI. Here’s a step-by-step guide to creating a list with swipeable items, complete with the definition of items
.
import SwiftUI
struct ContentView: View {
@State var items = ["Apple", "Banana", "Cherry", "Date"]
var body: some View {
List{
ForEach(items, id: \.self) { item in
Text(item)
.swipeActions(edge: .trailing, allowsFullSwipe: true) {
Button(role: .destructive) {
deleteItem(item)
} label: {
Label("Delete", systemImage: "trash")
}
}
.swipeActions(edge: .leading) {
Button {
pinItem(item)
} label: {
Label("Pin", systemImage: "pin")
}
.tint(.orange)
}
}
}
}
func deleteItem(_ item: String) {
items.removeAll { $0 == item }
}
func pinItem(_ item: String) {
print("\(item) pinned")
}
}
Key Components Explained
List and ForEach: The
List
andForEach
structures handle the display of items dynamically. Each item in the array can be interacted with through swipe actions.Swipe Actions: Swipe actions are added to each list item. You can customize actions for both the leading and trailing edges.
Handling Actions: The
deleteItem
function removes an item from the list, while thepinItem
prints a simple message. These functions are triggered by the corresponding swipe actions.
Advanced Features
- Conditional Swipe Actions: Sometimes, you might want to show actions conditionally based on the item’s properties. You can incorporate logic within your swipe actions to render them conditionally.
.swipeActions {
if item.isPinnable {
Button {
pinItem(item)
} label: {
Label("Pin", systemImage: "pin.fill")
}
.tint(.blue)
}
}
- Feedback and Animation: To make swipe actions feel more interactive, SwiftUI allows the addition of haptic feedback and animations to make the user experience richer.
Best Practices
Clarity and Functionality: Keep swipe actions relevant and limited to avoid clutter. Each action should be intuitive and clearly represented by an icon or text.
Accessibility and Testing: Ensure that all users can perform actions through alternative means. Test on various devices to guarantee consistent behavior.
Performance: Manage the list’s state efficiently to maintain smooth interactions, especially with large datasets.
Implementing swipe actions in SwiftUI is straightforward and enhances user interaction by providing quick and easy ways to perform common tasks. By integrating swipe gestures, you make your app more engaging and efficient, significantly improving the user experience.