Overview
SwiftUI’s Table view provides a powerful and flexible way to create multi-column lists, enabling developers to present data in a structured, tabular format. This article explores how to implement and use the Table view in SwiftUI to display data in multiple columns efficiently.
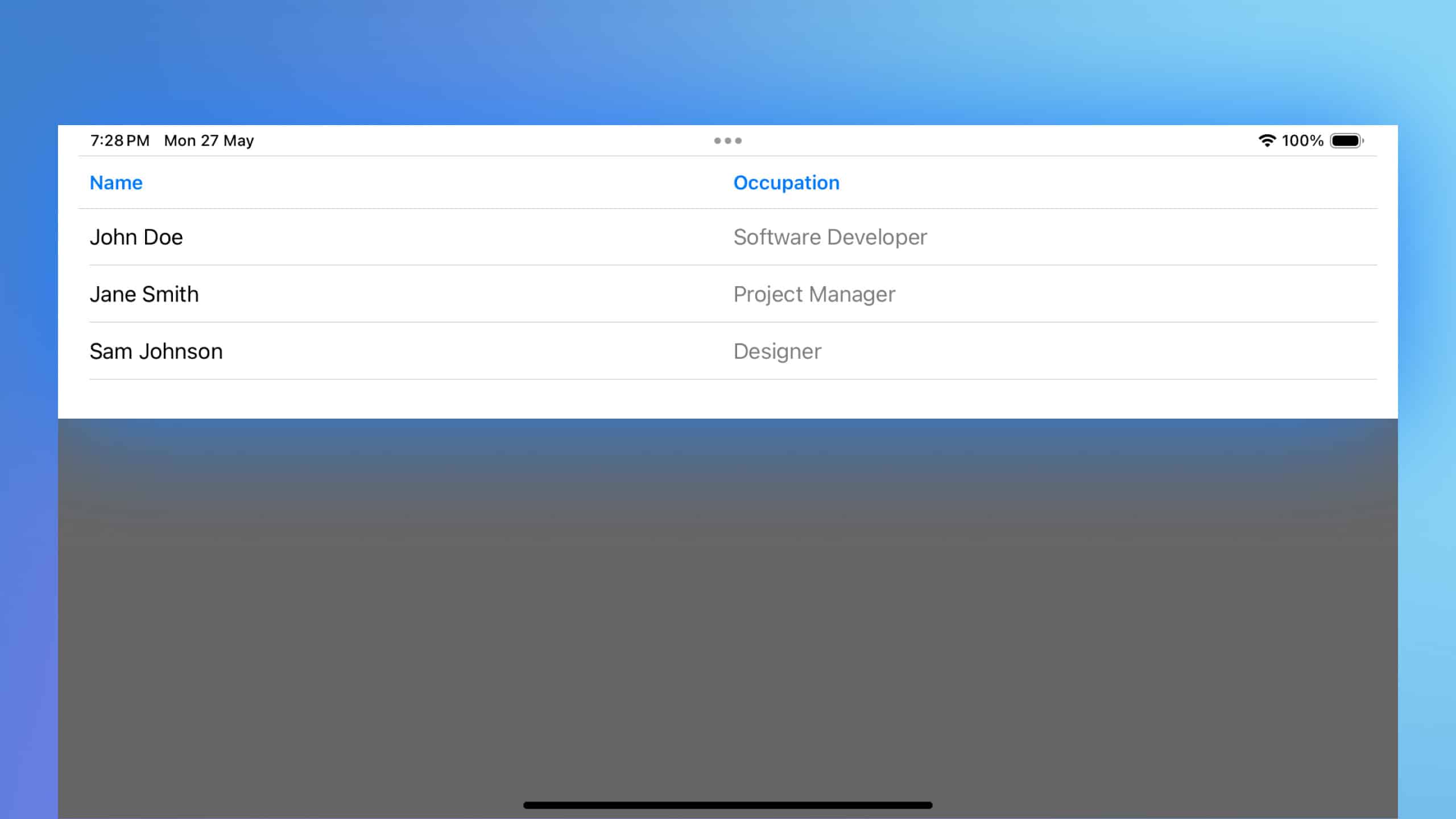
Code Snippet
import SwiftUI
struct Employee: Identifiable {
let id = UUID() // Unique identifier for each employee
let name: String
let occupation: String
}
struct ContentView: View {
let data = [
Employee(name: "John Doe", occupation: "Software Developer"),
Employee(name: "Jane Smith", occupation: "Project Manager"),
Employee(name: "Sam Johnson", occupation: "Designer")
]
var body: some View {
Table(data) {
TableColumn("Name", value: \.name)
TableColumn("Occupation", value: \.occupation)
}
}
}
Code Explanation
struct Employee
: Defines a structure for employee data that includes unique identifiers (id
), names, and occupations. This struct conforms to theIdentifiable
protocol, which is necessary for using each instance in a SwiftUITable
.Table(data) { ... }
: Constructs a table using the array ofEmployee
objects. EachEmployee
is represented in the table with rows that bind to thename
andoccupation
properties.TableColumn("Name", value: \.name)
: Specifies a table column for employee names.TableColumn("Occupation", value: \.occupation)
: Specifies another column for displaying the occupation of each employee.
Using Table
in SwiftUI provides a straightforward way to create multi-column lists, making it easier to present complex data in a readable and organized format. This feature is particularly useful for iPadOS
and macOS
applications that require displaying tabular data, such as contact lists, data reports, or any scenario where structured information is essential. By leveraging SwiftUI’s declarative syntax, developers can quickly implement and customize tables to suit their app’s needs.