Overview
This article provides an in-depth look at the Text
view in SwiftUI, one of the most essential components used for displaying read-only text in iOS applications. We’ll cover how to customize text with various modifiers to adjust its font, color, alignment, and more, enhancing the visual appeal and functionality of your SwiftUI views.
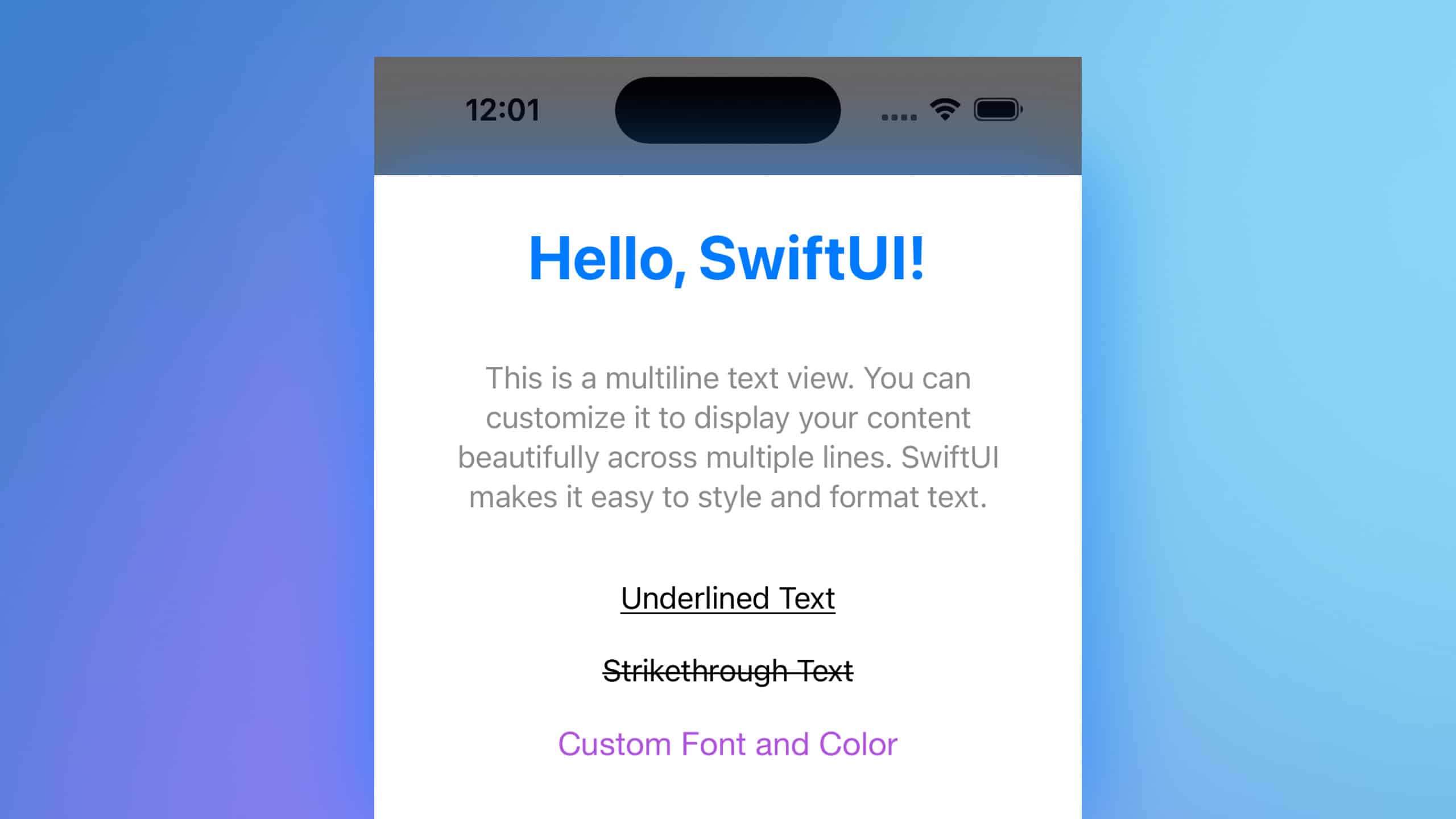
Code Snippet
import SwiftUI
struct ContentView: View {
var body: some View {
VStack(spacing: 20) {
Text("Hello, SwiftUI!")
.font(.largeTitle)
.fontWeight(.bold)
.foregroundStyle(.blue)
Text("This is a multiline text view. You can customize it to display your content beautifully across multiple lines. SwiftUI makes it easy to style and format text.")
.font(.body)
.foregroundStyle(.secondary)
.multilineTextAlignment(.center)
.lineLimit(nil)
.padding()
Text("Underlined Text")
.underline()
Text("Strikethrough Text")
.strikethrough()
Text("Custom Font and Color")
.font(.custom("Helvetica Neue", size: 18))
.foregroundStyle(.purple)
}
.padding()
}
}
Code Explanation
VStack(spacing: 20)
: Creates a vertical stack with 20 points of spacing between each element.Text("Hello, SwiftUI!")
: Displays a single line of text..font(.largeTitle)
: Sets the font size to large title..fontWeight(.bold)
: Makes the text bold..foregroundStyle(.blue)
: Sets the text color to blue.
Text("This is a multiline text view...")
: Displays a multiline text..font(.body)
: Sets the font size to body..foregroundStyle(.secondary)
: Uses a secondary color for the text..multilineTextAlignment(.center)
: Aligns the text in the center..lineLimit(nil)
: Allows unlimited lines for the text..padding()
: Adds padding around the text.
Text("Underlined Text")
: Displays underlined text..underline()
: Underlines the text.
Text("Strikethrough Text")
: Displays text with a strikethrough..strikethrough()
: Adds a strikethrough to the text.
Text("Custom Font and Color")
: Displays text with a custom font and color..font(.custom("Helvetica Neue", size: 18))
: Sets a custom font and size..foregroundStyle(.purple)
: Sets the text color to purple.
The Text
view in SwiftUI is highly versatile and can be easily customized to fit the needs of your application. By leveraging various modifiers, you can control the appearance and behavior of your text, ensuring it aligns with the design and functionality of your app. Experiment with these modifiers to create visually appealing and user-friendly interfaces.