Overview
This article demonstrates how to integrate a WKWebView into a SwiftUI view, allowing you to display web content within your iOS application. WKWebView is a powerful tool from the WebKit framework that can render web pages.
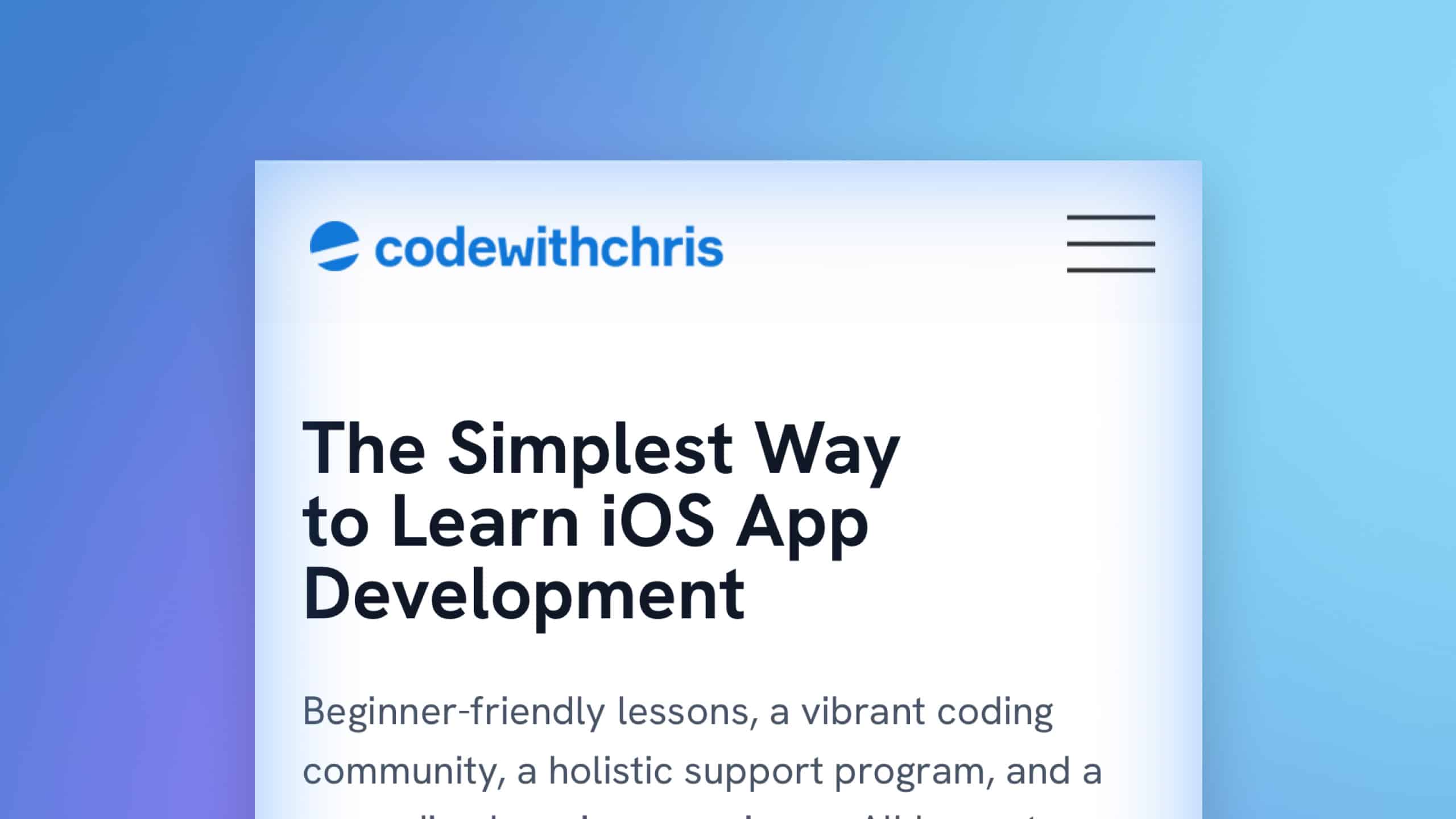
Code Snippet
import SwiftUI
import WebKit
struct WebView: UIViewRepresentable {
let url: URL
func makeUIView(context: Context) -> WKWebView {
return WKWebView()
}
func updateUIView(_ webView: WKWebView, context: Context) {
let request = URLRequest(url: url)
webView.load(request)
}
}
#Preview {
WebView(url: URL(string: "https://codewithchris.com/")!)
.edgesIgnoringSafeArea(.all)
}
Code Explanation
- Import WebKit – The
import WebKit
statement is necessary because it provides access to the WKWebView class, which is part of the UIKit framework and not included in SwiftUI by default. This import is essential for embedding web content in SwiftUI views. - WebView struct – This struct conforms to
UIViewRepresentable
, which is necessary to integrate any UIKit component into SwiftUI. It takes aURL
as a parameter.makeUIView
: Creates and returns aWKWebView
instance. This method initializes the web view.updateUIView
: Loads the provided URL into the web view. This is where the web content is set up to display.
- How to use it? To use our WebView, start by initiating it in our preview. This process involves calling the WebView and passing a specific URL to it. To ensure the WebView extends into the safe areas of the display, apply the
.edgesIgnoringSafeArea(.all)
modifier. This concept can also be applied if you decide to use our WebView in other views.
struct ContentView: View {
var body: some View {
WebView(url: URL(string: "https://codewithchris.com/")!)
.edgesIgnoringSafeArea(.all)
}
}
Integrating WKWebView within SwiftUI views provides seamless web content integration in your iOS apps. By using UIViewRepresentable
, you can leverage existing UIKit components effectively in a SwiftUI context, ensuring you can use a vast array of features not natively supported by SwiftUI yet. This setup is ideal for displaying interactive web content directly in your applications.