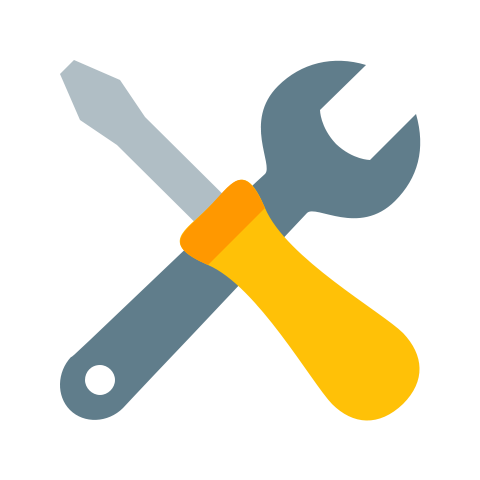
Are you getting strange error messages like “Thread 1: signal sigabrt” or “Use of unresolved identifier”?
This handy reference guide will show you what the common causes for these errors are and give you steps to fix them!
TABLE OF CONTENTS
1. Xcode Simulator Errors
– My iPhone simulator looks different?
– My simulator screen is black when the app runs
2. App Runs And Then Crashes
– Finding the error message after a crash
– Unrecognized selector sent to instance
– This class is not key value coding-compliant for the key…
– Unexpectedly found nil while unwrapping an optional value
– Thread 1: signal sigabrt
– Use of unresolved identifier
– Class has no initializers
3. Xcode Errors/App Won’t Run
– Xcode complains that a method of some class doesn’t exist
– Xcode warnings
4. My Xcode Interface Is Different
– Missing panels or views
– Can’t find UIElements in my Storyboard
– Can’t drag UIElements onto my Storyboard
1. Xcode Simulator Errors
My iPhone simulator looks different?
If your iPhone simulator doesn’t look like the one you see me using in my videos, it’s because your iOS simulator is at a different zoom level.
The frame or bezel on the simulator also differs from level to level so if you don’t see an iPhone bezel on your simulator, try changing your zoom level to either “Physical Size,” “Point Accurate,” or “Pixel Accurate” as shown in the screenshot below. By default, the zoom level is “Physical Size.”
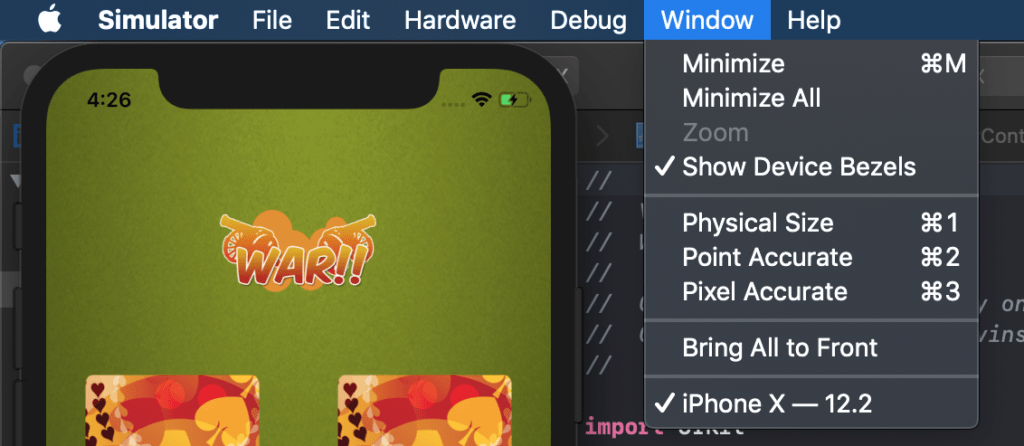
You can also hit command + 1-3 on your keyboard as shortcut keys to control the zoom level of your simulator.
My simulator screen is black or white when the app runs
If you run your app and the iOS simulator is showing a black or white screen, I would first wait a little while. Sometimes it can take up to 15 seconds to launch your app initially.
Secondly, I would reset the simulator like I mentioned above and try to run my app again.
If that still doesn’t work, then maybe you have accidentally set some breakpoints which is stopping the app execution.
Run your app, then bring Xcode to the foreground and see if you see something like the below with a green line (representing where execution has halted) stopped at a blue marker (which is a breakpoint):
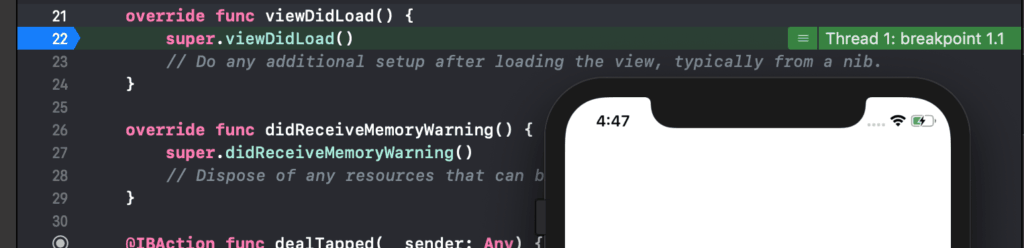
If you see something like the above screenshot, that means that you’re seeing a black screen because the app execution has stopped. All you need to do is to either remove the breakpoint by clicking the blue marker and dragging it off the gutter (and then re-run your app).
Or you can disable all breakpoints by this menu command in Xcode:
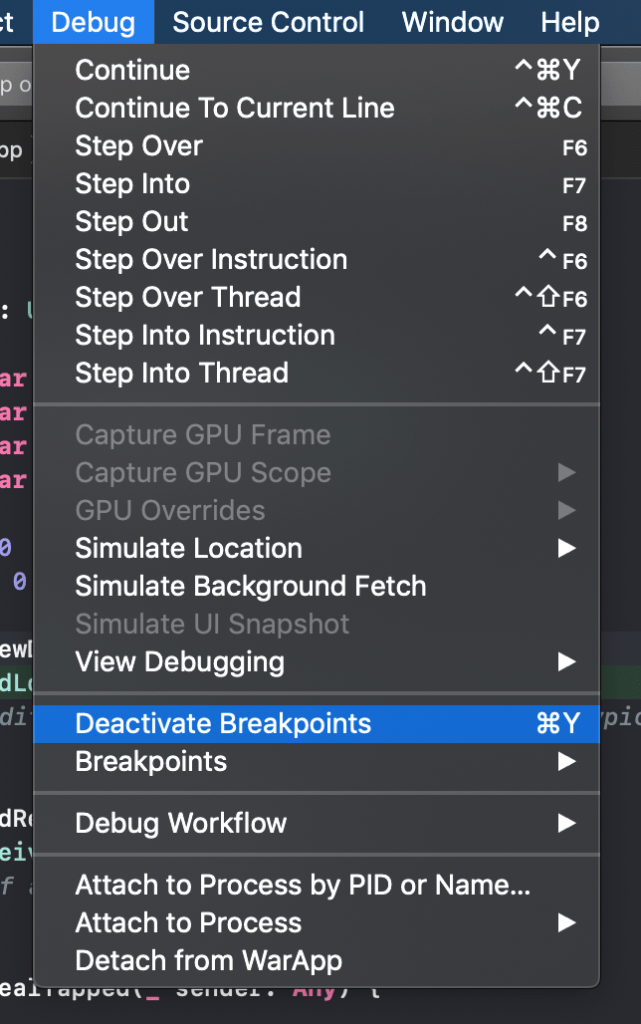
I see this happening a lot for beginners who aren’t aware of what breakpoints are.
Breakpoints are used to halt execution at a certain point so that you can inspect variables and peek into your objects to debug.
You can set breakpoints by clicking the gutter beside a line of code and it’ll add a little blue marker there. So you can see why beginners may accidentally have set breakpoints and not realize it when they run their app!
2. App Runs And Then Crashes
Finding the error message after a crash
When your app runs and then crashes (as soon as it runs or after you click something), you need to know where to find the error message to understand exactly what went wrong.
After the crash, go to Xcode in the lower right hand pane, scroll all the way to the top.
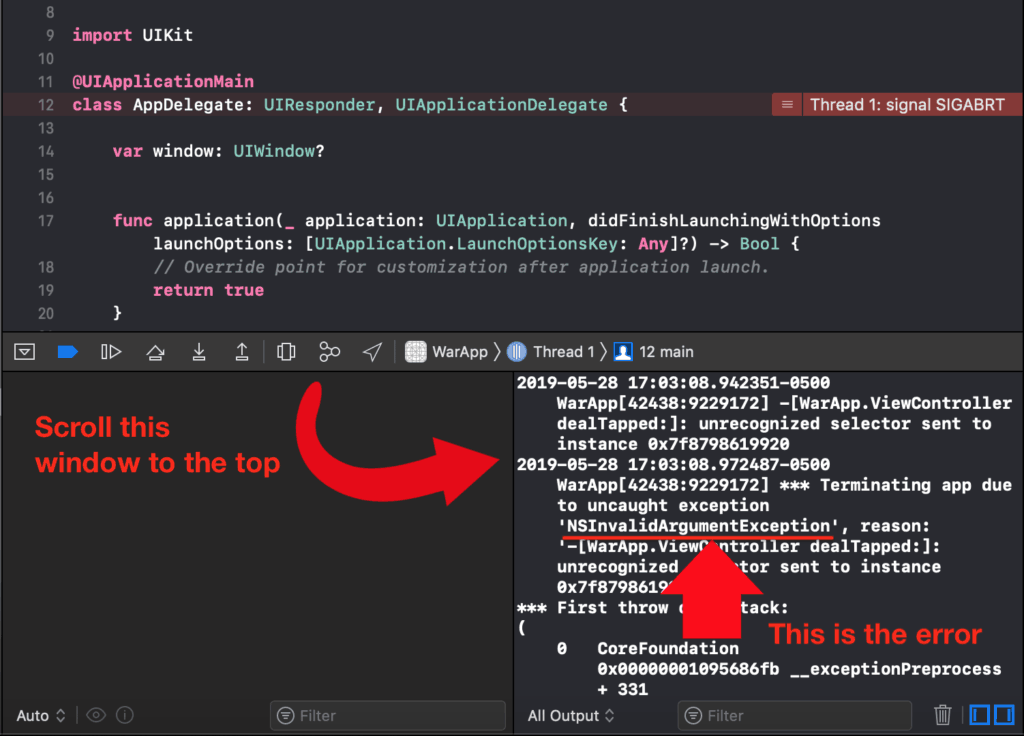
Unrecognized selector sent to instance error
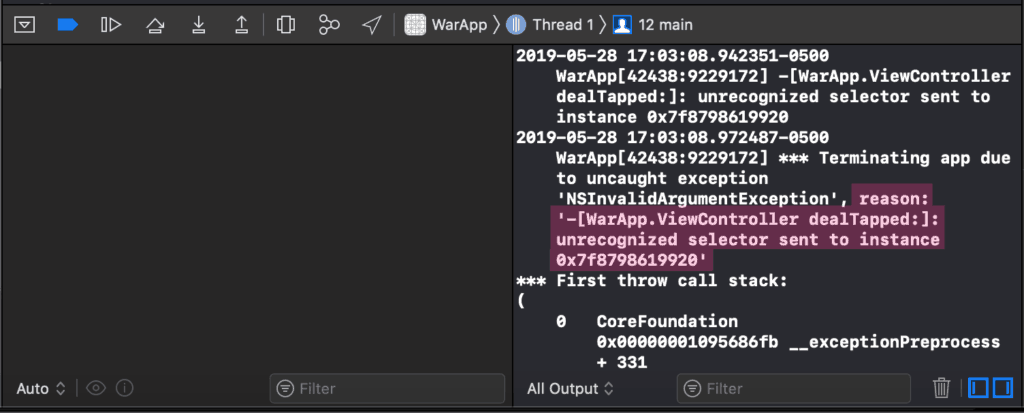
So now that you know how to find the actual error message when your app crashes, the next step is to learn to recognize some of the more common types of messages because they will hint at what’s wrong.
The unrecognized selector error means that somewhere, a method was called on an object and that object didn’t have that method so it crashes. “Selector” is another term for a method.
As you can see in the error message above, it tells you the method that was called was “dealTapped” and the object that it was called on was a ViewController object.
So now you can go back to your code and see where you’re calling the “dealTapped” method and why the object doesn’t have that method.
Check if that method exists in the .swift file of the class and pay attention to the parameters and letter casing to make sure it matches.
If you’re calling a method on a IBOutlet property that is referring to an element in your storyboard, then make sure that the UIElement has its custom class set to the class that has the “dealTapped” method.
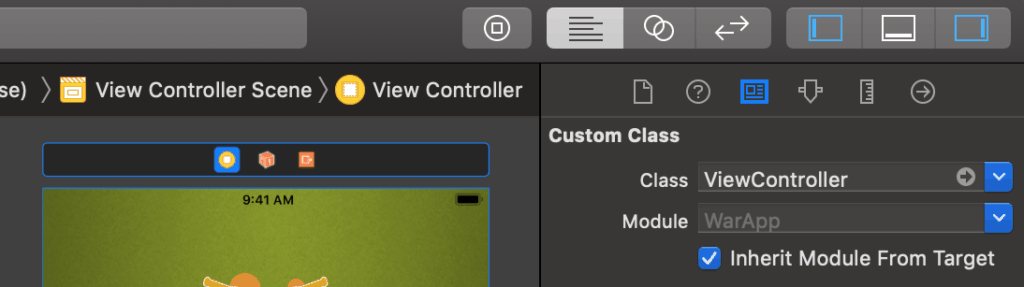
This class is not key value coding-compliant for the key
If you’re using Storyboards to build your user interface and you encounter a detailed error similar to this:
*** Terminating app due to uncaught exception ‘NSUnknownKeyException’, reason: ‘[setValue:forUndefinedKey:]: this class is not key value coding-compliant for the key handleButtonClick.’
The actual name of the key (in this case “handleButtonClick”) may be different for you, but this error may indicate that there’s a UIElement in your Storyboard that’s connected to a property which doesn’t exist anymore. This can occur if you connected the UIElement to a property and then removed the property from the .swift file.
In order to fix it, go into your storyboard and right click each element to check the connections to the properties and “break” any connections to properties that don’t exist in your .swift file anymore by clicking the little “x” beside each bad connection.
Unexpectedly found nil while unwrapping an optional value
Another one of the most common errors is the “unexpectedly found nil while unwrapping an optional value” error.
This error usually occurs after attempting to use a nil
value as a regular value. For example, if you force unwrap an optional value with the “!” operator since you expect it to contain a non-nil
value, receiving this error indicates that the optional value actually contained nil
.
In the screenshot below, I attempt to compare the value of str
with “CodeWithChris” to see if they are equal. However, I receive this error because str
contains nil
.
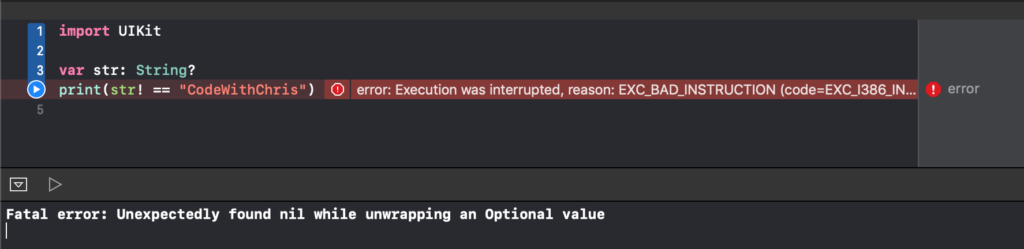
This error can also be caused by attempting to use disconnectedIBOutlet
properties. IBOutlet
properties are actually implicitly unwrapped optionals, which means that they start off as nil
but are expected to contain a value when your app runs. This can cause problems if you disconnect an IBOutlet
in your storyboard but still refer to it in your code.
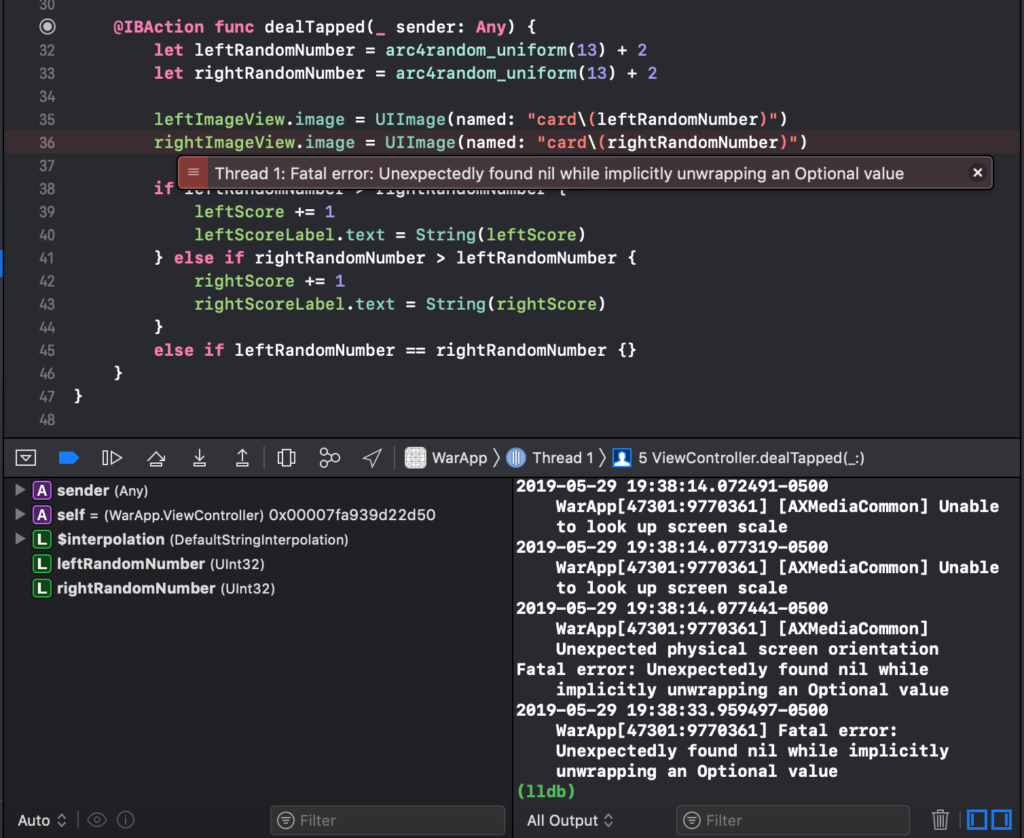
Thread 1: signal sigabrt
This unhelpful error usually appears next to the red highlighted line when Xcode crashes. Because this error does not give you specific information about what’s wrong with your app, I would recommend looking for the detailed error as discussed above.
The signal sigabrt message could be caused for several reasons, including attempting to dequeue a TableView cell with an identifier that does not exist (as seen in the screenshot below) or attempting to perform a segue with an identifier that does not exist.
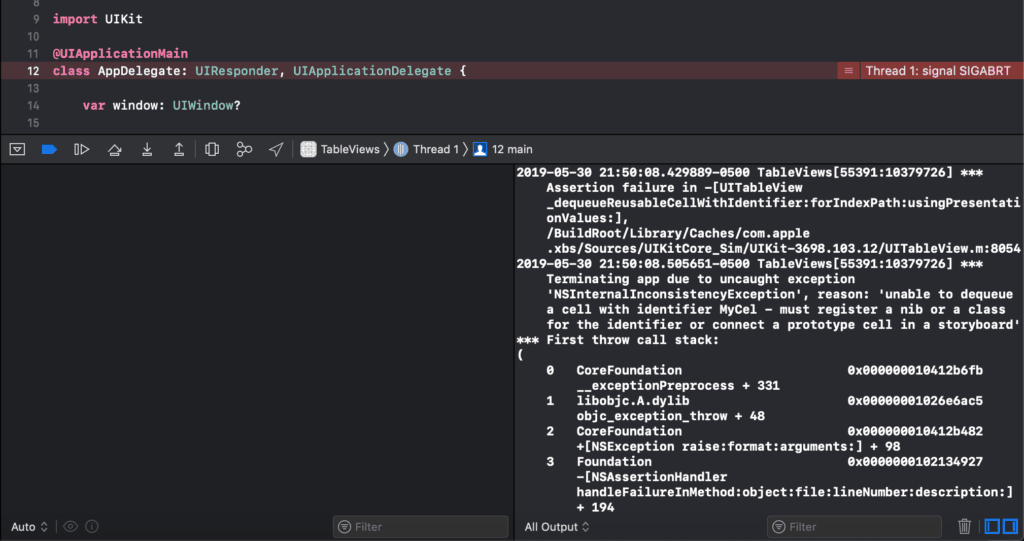
In these cases, double check to make sure that you have not made any typos when typing out your identifiers. Otherwise, you may have to Google the detailed error message for a better idea of the cause of the problem.
Use of unresolved identifier
You will often see this error if Xcode cannot find the variable or property you are referring to. “Unresolved” means that Xcode couldn’t find something, and “identifier” is another word for a variable, property, function name, etc.
Here are several reasons why you could receive this error:
- You made a typo when trying to access a variable or property.
As you can see in the screenshot above, I declare a variable calledname
and set it equal to David. On the next line, I attempt to print outname
. However, I receive an “unresolved identifier” error because I typednome
instead ofname
. - You are trying to access a property out of scope.
Variable scope is something that often confuses beginners. In the screenshot above, I have a function calleddoWork()
. Inside the function, I declare aString
constant calleddoingWork
, and I am able to print it inside the function.
However, if I try to printdoingWork
outside thedoWork
function, I receive the “unresolved identifier” error. This is because I can only accessdoingWork
inside the scope of the function. - You have not imported a method or framework where a property is declared.
You will often receive this error if you forgot to import a library or framework. In order for the code in the screenshot above to work, I need to make sure to importUIKit
to get access toarc4random_uniform
andUIImage
.
Class has no initializers
This is another error that often confuses beginners.
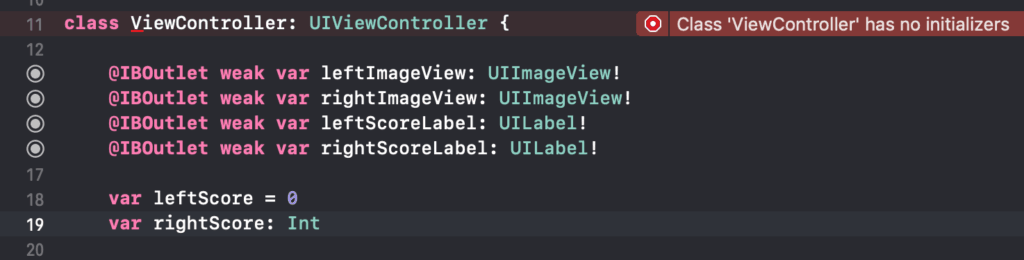
To explain this error, let’s take a look at the screenshot above, specifically at the leftScore
and rightScore
variables. In order for a class in Swift to be initialized, Xcode needs to guarantee that all of its non-optional properties will also be initialized.
This is the reason why we are seeing the “no initializers” error. Because rightScore
does not have an initial value as leftScore
does and because the ViewController
class does not have an init()
method, Xcode cannot guarantee that leftScore
will be initialized when the ViewController
is.
If you are a beginner, I would recommend two solutions to fixing this error. You can either give rightScore
an initial value similar to leftScore
, or you can make rightScore
optional.
You can also give your view controller class an initializer. However, this is more of an advanced topic since it involves making an instance of your ViewController
programmatically.
3. Xcode Errors/App Won’t Run
Xcode complains that a method of some class doesn’t exist
If you run your app and you get some build errors, that means Xcode has detected that the syntax of your code is wrong.
If Xcode is complaining that a specific identifier doesn’t exist, then check that all of your opening curly braces have corresponding closing curly braces.
If you look at the screenshot below, both build errors are caused because of missing curly braces. Can you spot the missing curly brace?
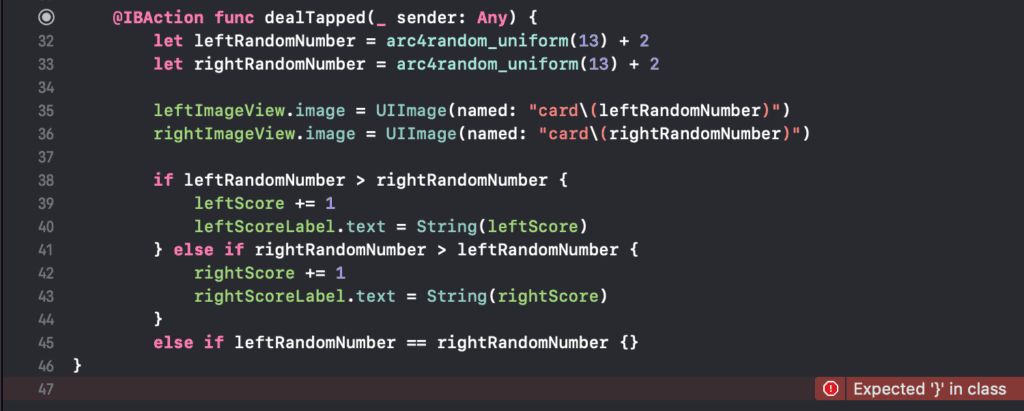
Xcode warnings
Xcode warnings are highlighted in yellow and they won’t stop you from running your app but it’s a good practice to reduce the number of them.
I’ll be illustrating some of the more common ones in the future in this section.
4. My Xcode Interface Is Different
Missing panels or views
If you’re missing some panes in your Xcode interface, you can check the buttons in the upper right corner. They’re used to toggle the main panels.

In the debug/console area, there are also a couple of buttons to toggle the panes in the debug area:
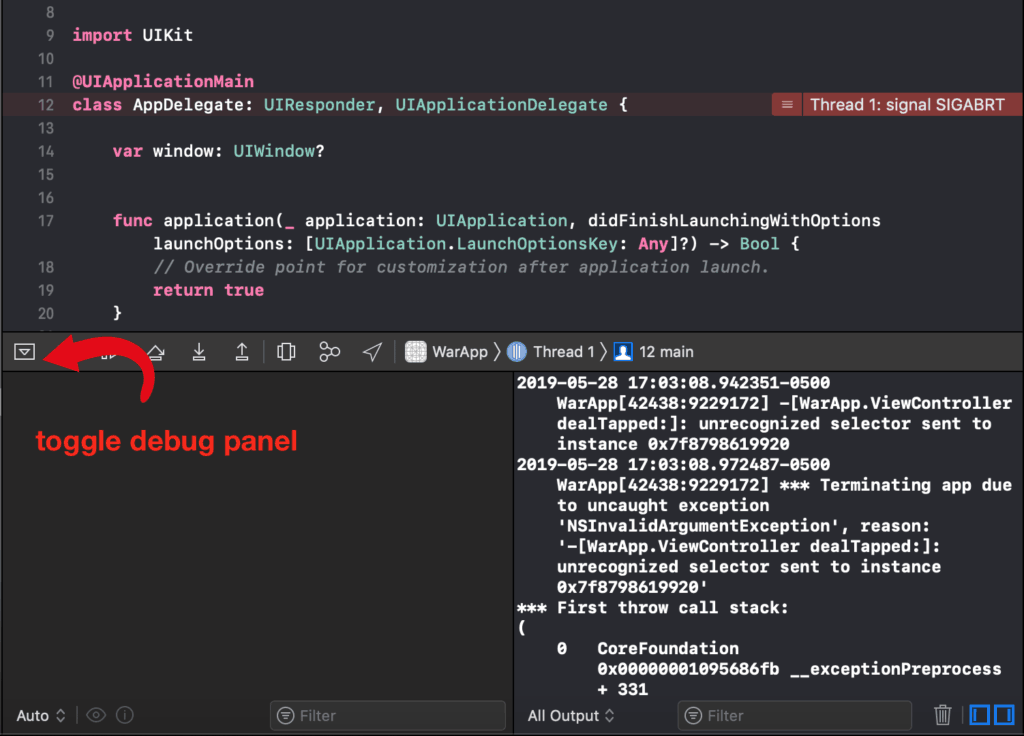
If you’re in Storyboard designer view, there’s a button that many people miss. This button controls the document outline which lists the UI Elements in your storyboard in a tree hierarchy view.
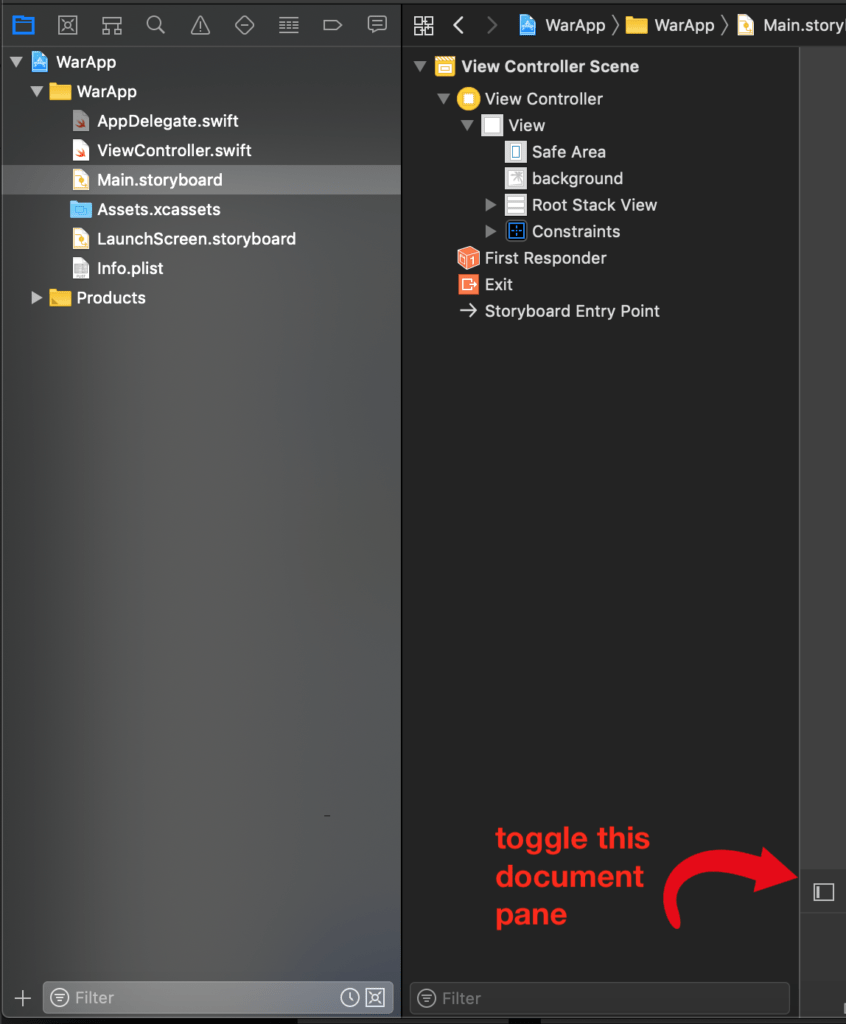
Can’t find UIElements in my Storyboard
If you don’t see the UI elements list in your storyboard view then your library pane may not be visible. See the screenshot below:
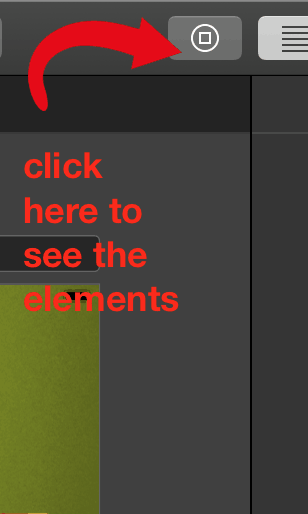
Can’t drag UIElements onto my Storyboard
If you drag UI elements onto your storyboard and you can’t add any to your view, then you may be in zoomed out view. When you’re zoomed out in your storyboard, you can’t add any elements into the view. The zoomed out view gives you and overview of your views and allows you to position your controllers on the storyboard.
To get back into zoomed in view, just double click on an empty area on your storyboard and you’ll zoom back in and be able to drag elements onto your views.