In this tutorial, you’re going to get a tour of Xcode 11 and an introduction on how to get started with Apple’s new framework called SwiftUI.
You’ll learn how to set up an Xcode 11 Swift UI project, how to create new views, combine existing views using VStack
and HStack
, and finally, how to use the preview canvas to see how your user interface looks.
1. Xcode 11 Set Up
Before we get started, there are a few things that you should be aware of.
- You can install Xcode 11 for free through Apple’s developer website. If you are running macOS Mojave, you can use SwiftUI without the live preview and design canvas features. If you want to use these features, you will need to install the beta version of macOS Catalina which requires a paid developer account.
- Note: beta software is not always stable, so use it at your own risk.
- Once you have Xcode 11 installed, you can switch between Xcode 11 and Xcode 10.
Let’s begin by starting a new Xcode project in Xcode 11. Remember to check “Use SwiftUI” in the project creation window.
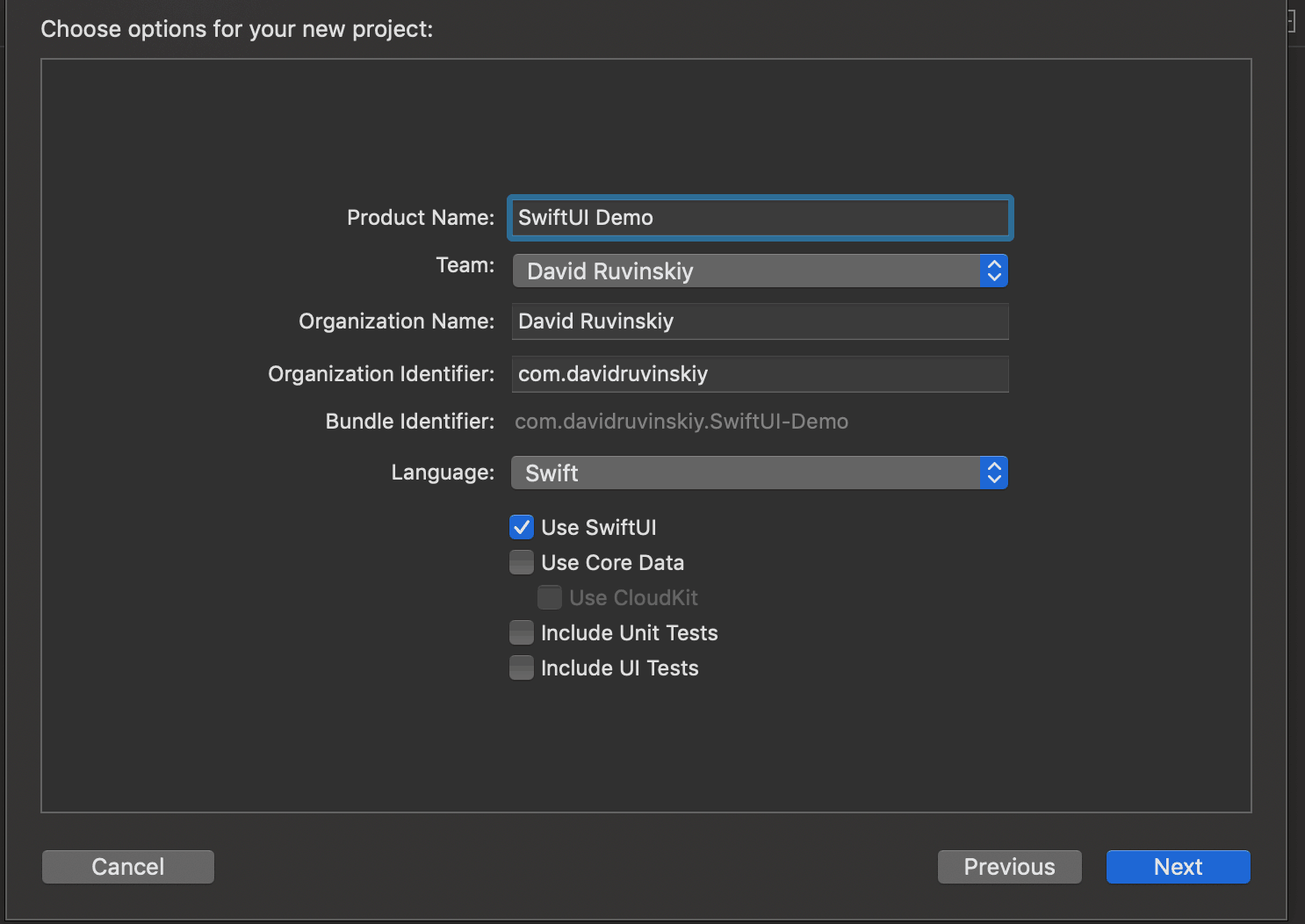
2. Xcode Tour
Now, let’s take a look at the ContentView.swift
file. If you’re used to using UIKit, this is similar to the ViewController.swift
file which is created by default when you start a new Xcode project.
Once your project is created, you should see a screen that looks like this:
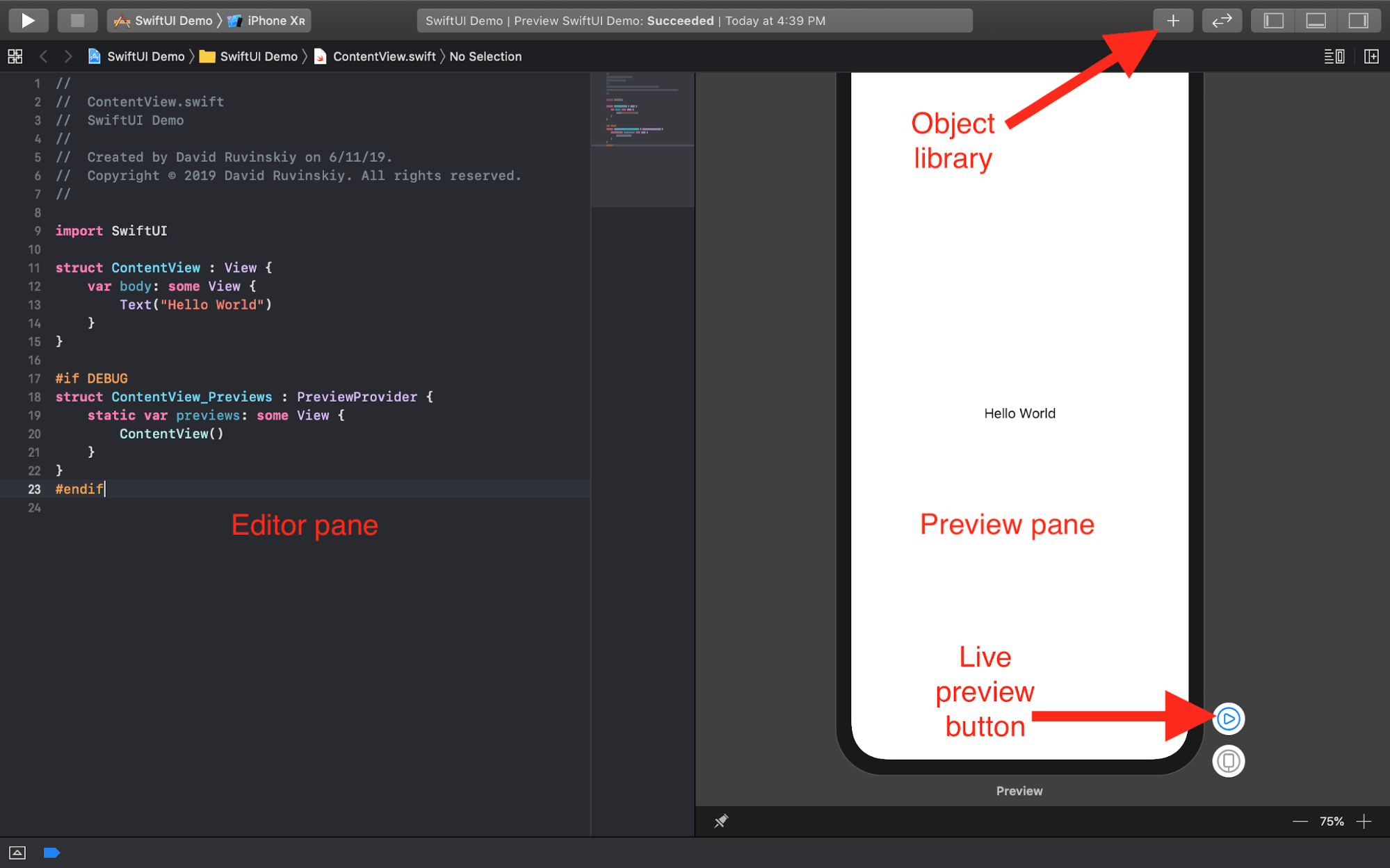
Take note of the editor pane, preview pane, object library, and live preview button in the preview pane. You may also see a resume button in the preview pane if you make a large change in your code and Xcode is waiting for confirmation to display the changes.
Let’s take a closer look at the editor pane:
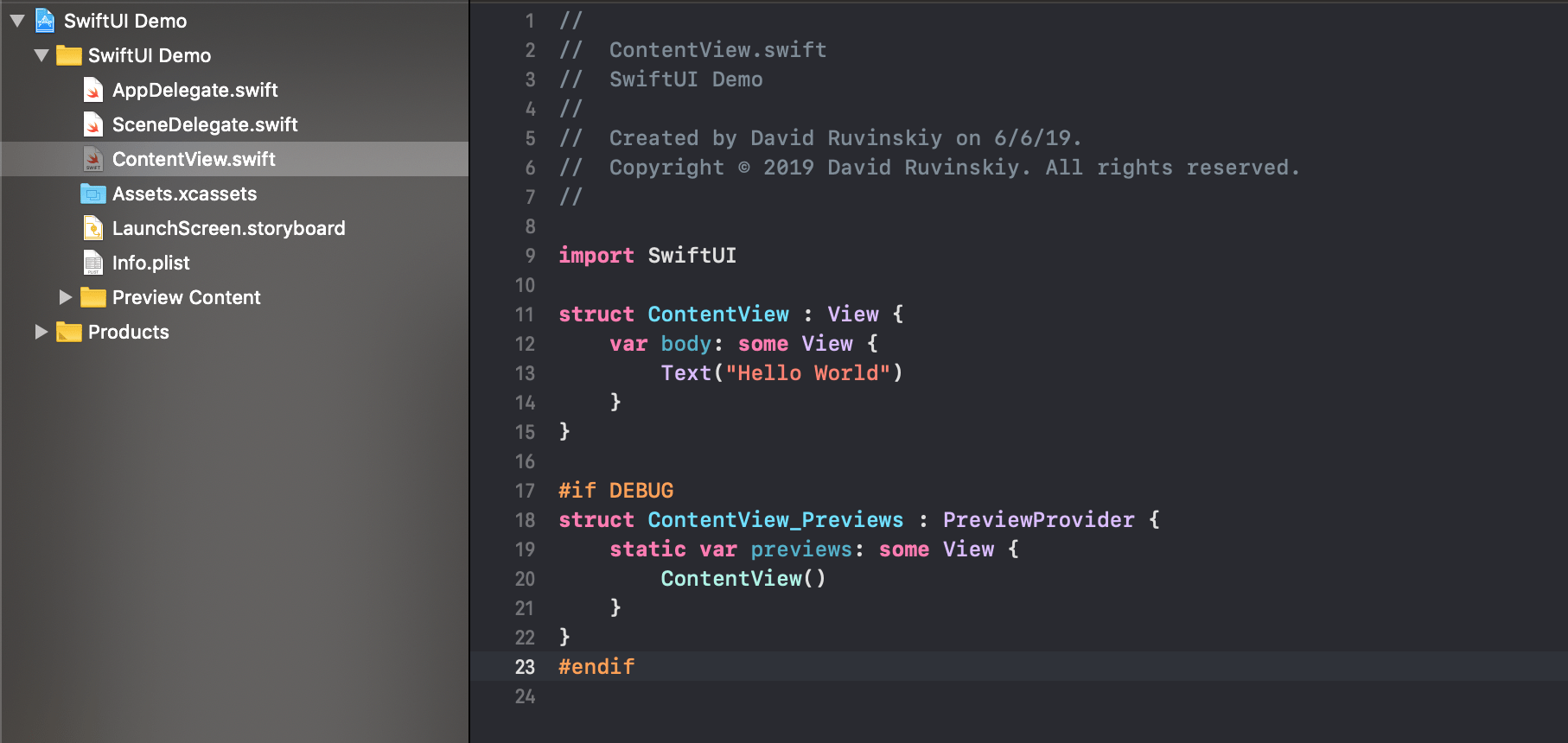
You may be wondering why we have a ContentView.swift
file created at all. To answer that, let’s go into the SceneDelegate.swift
file and scroll down to the willConnectTo session
method.
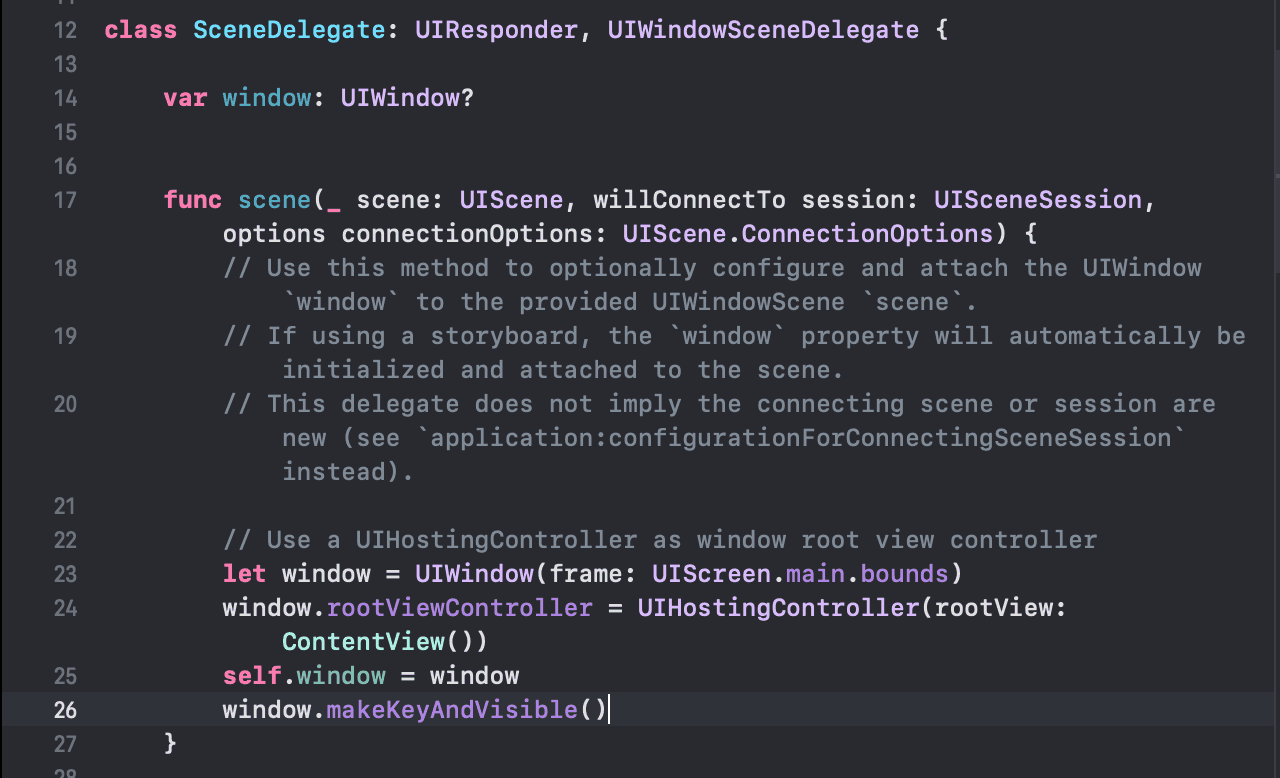
As you can see, SceneDelegate.swift
is creating an instance of ContentView
and is setting it as the root view.
3. The ContentView and Preview
Now, let’s take a closer look at the ContentView.swift
file. This contains two structs: ContentView
and ContentView_Previews
. The ContentView
struct is responsible for the content and layout of your screen, while ContentView_Previews
is responsible for creating the preview that you see on the right side.
Something that you may notice when working with SwiftUI is that there is no Main.storyboard
file. This is because you can now design your layout by editing the code or editing the preview.
Whatever changes you make in code will update the preview, and whatever changes you make in the preview will update the code.
Try modifying the “Hello World” text view by holding down the command key, clicking on the text view, and clicking on the “Inspect” button in the menu that pops up. Notice that any changes you make in the preview also updates your code.
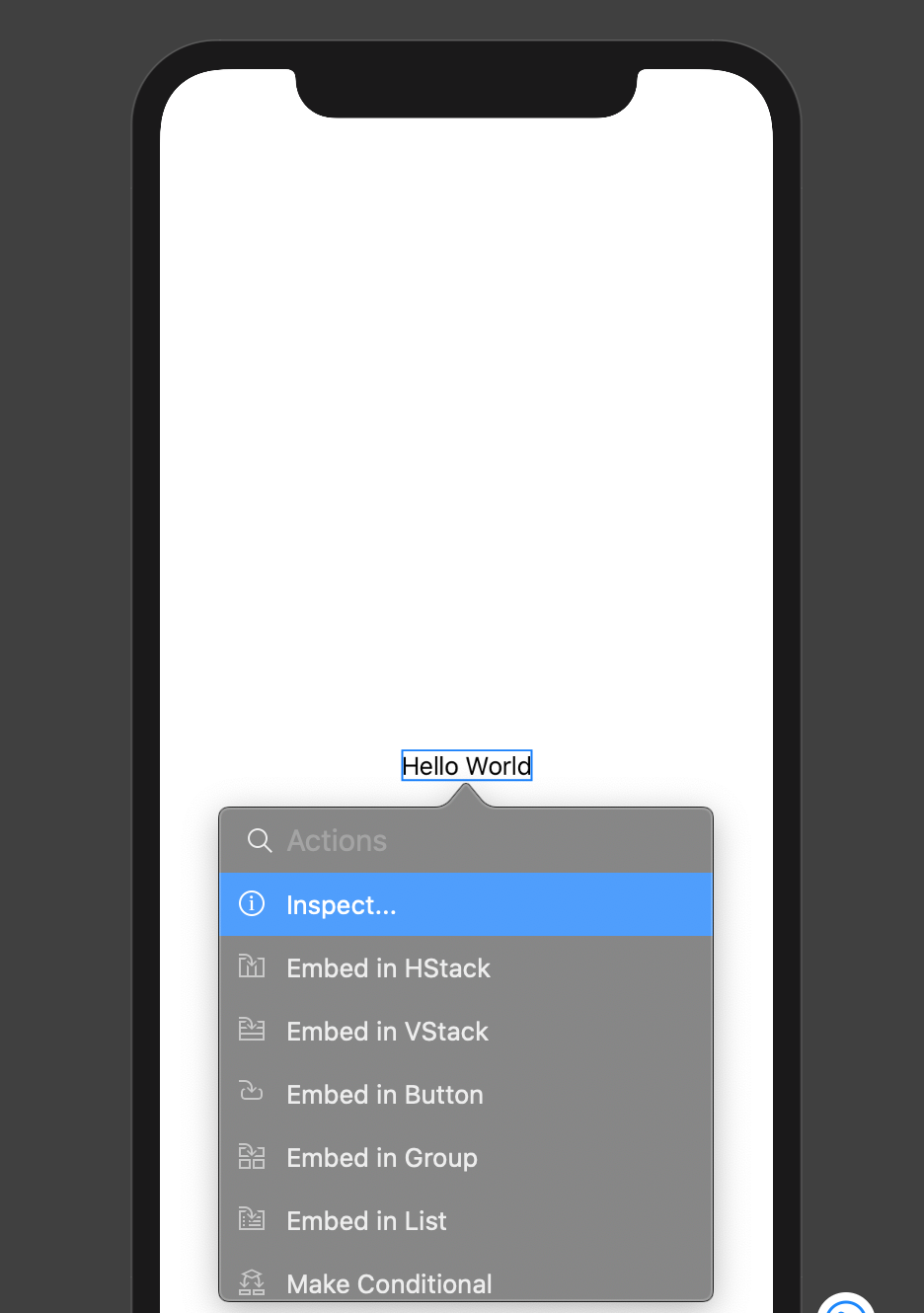
Note that if there is ever a discrepancy between the code and the preview, the code is always the source of truth since the preview is generated from the code.
4. Creating Views
Now, let’s take a look at creating a few view elements. In SwiftUI, anything that can be displayed on screen is considered a view and must conform to the View
protocol. If you want a class to conform to the View
protocol, it must provide a body
property as seen in the screenshot below.
Let’s try to replace the text view that’s currently displaying “Hello World” with an image:
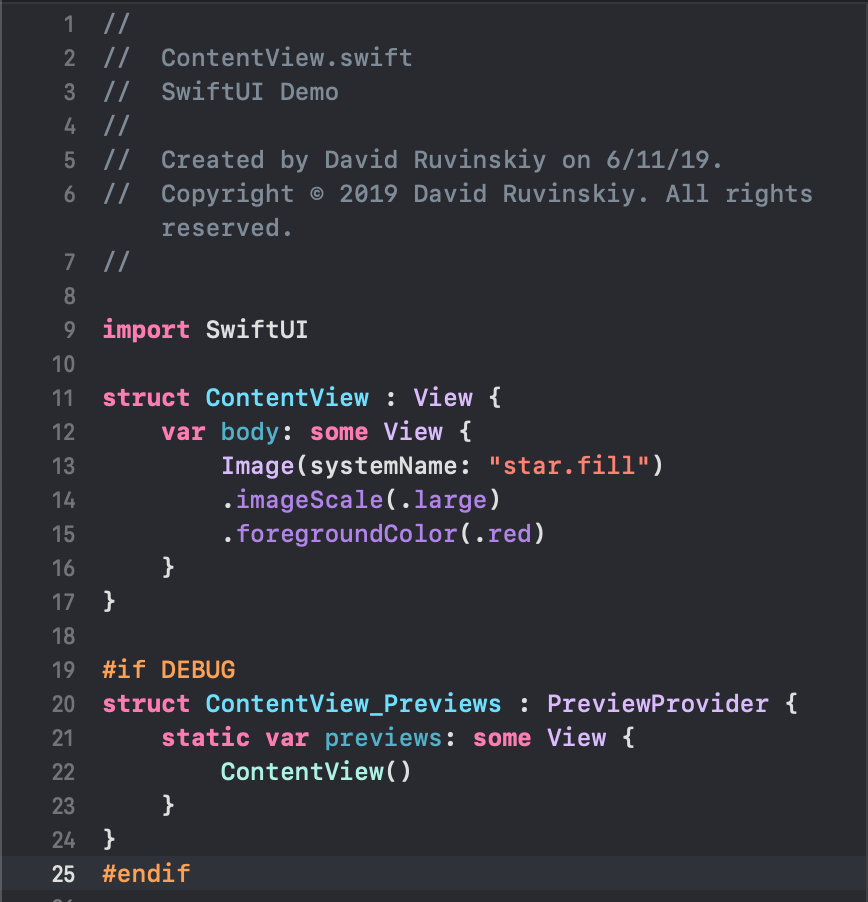
The code above creates an image using one of the icons Apple has provided developers in Xcode 11 and displays that image on screen.
5. View Modifiers
Now, you may be wondering what the .imageScale(.large)
and .foregroundColor(.red)
lines of code are doing. These are called modifiers, and they help to make SwiftUI declarative instead of imperative.
Whenever you create a view in SwiftUI, you can apply various modifiers to it to customize it. For example, the code above is telling SwiftUI to make the image large and to give it a foreground color of yellow.
6. Combining Views Together
If you want to see a list of all the view elements you can add to your screen, click the plus button in the top right corner of Xcode. If you want to add a particular element to your screen, you can do so by dragging and dropping from the object library directly into the editor.
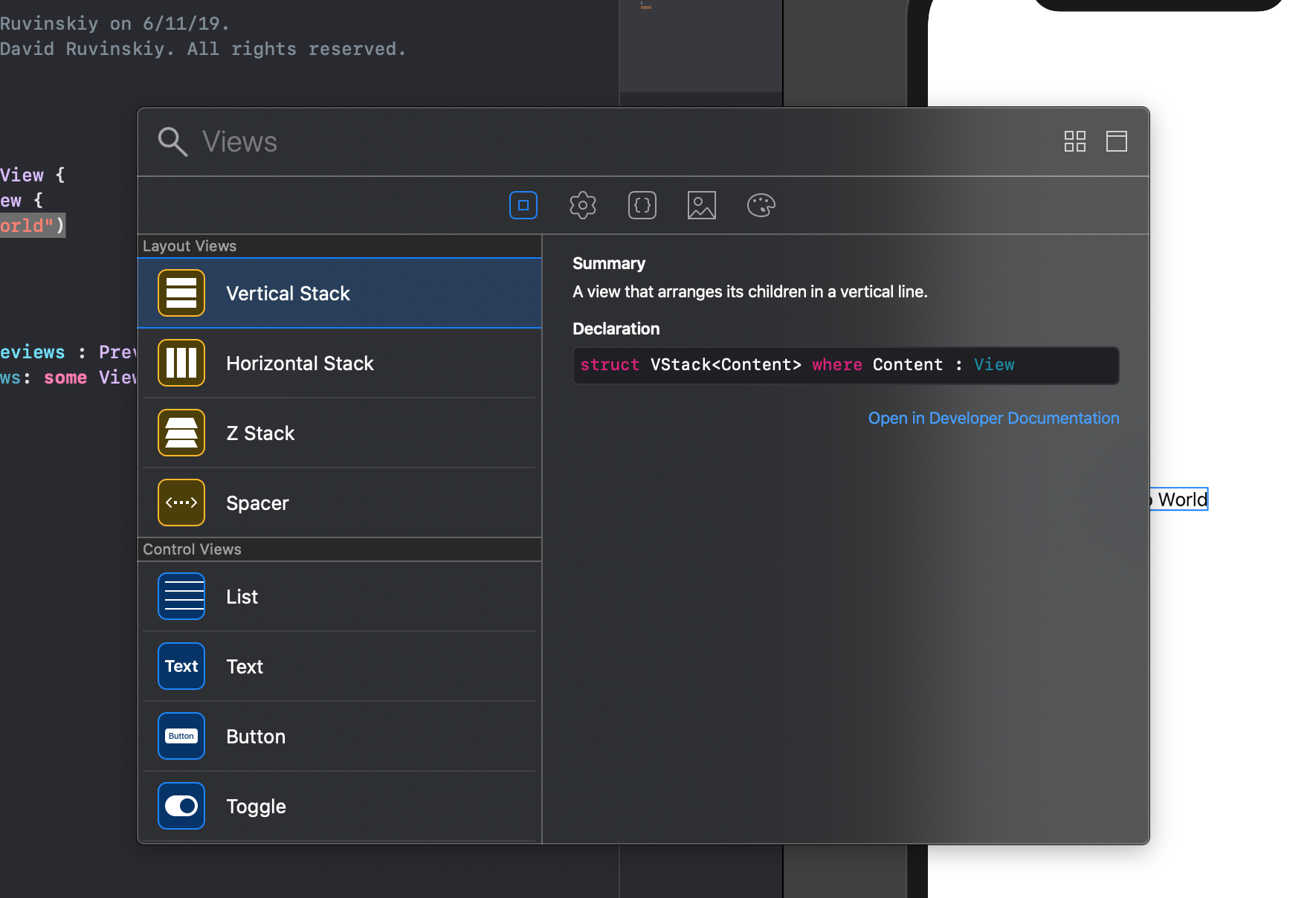
Let’s finish this lesson by discussing how you can combine views. SwiftUI comes with something called a VStack
and an HStack
which are similar to vertical and horizontal stack views in UIkit.
Let’s try adding two text views to the screen using a VStack
.
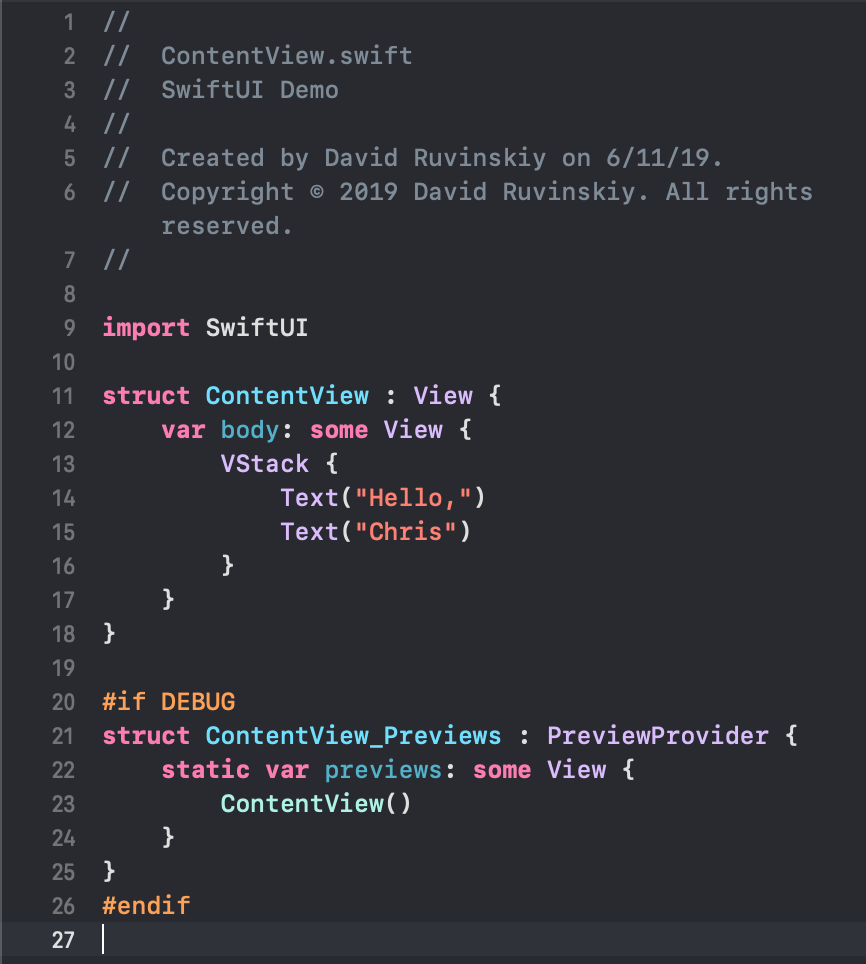
The code above will display “Hello,” above “Chris” on screen. If you want the text to be side by side, you can simply change the VStack
to an HStack
.
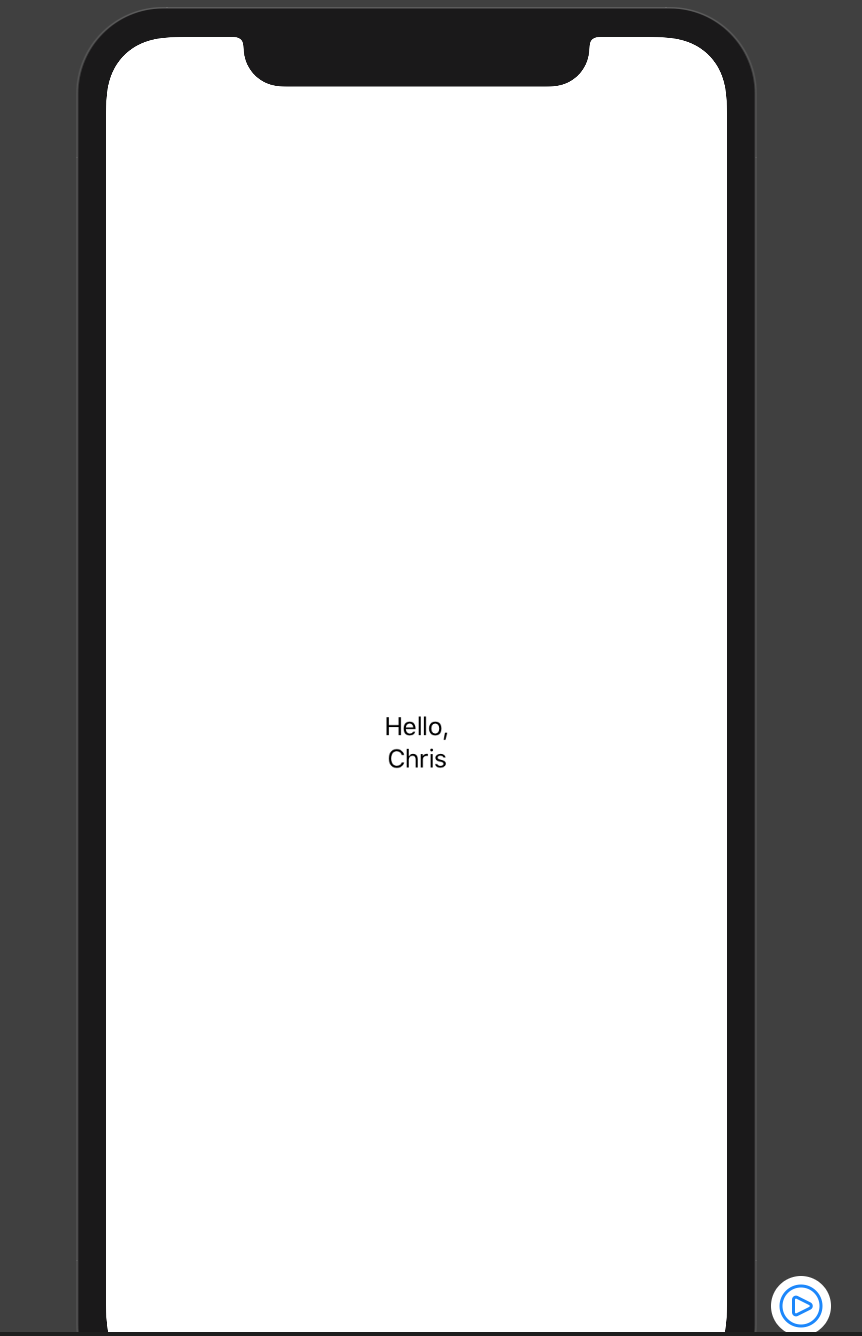