CodeWithChris IndexSwift and SwiftUI Tutorials
Hello and welcome!
Below you’ll find all of our training organized by category. We’re still in the process of indexing all of our content so please check back periodically!
Categories
Recommended Resources

14 Day Beginner Challenge
Follow this challenge to start your App Journey!

Learn Swift for Beginners
Learn swift programming even if you have no coding experience!

App Idea to Launch
Here are the 10 steps to go from app idea to app launch!

Xcode Tutorial For Beginners
My ultimate guide to using Xcode for beginners

Xcode for Windows
If you’re using a Windows PC, this guide will get you up and running with iOS App Development
CWC+ Courses Index
The course indexing is a new initiative for us and it’ll take some time to do this for each of courses so please bear with us as we work hard to bring you the indexes. For a complete listing of our courses, click here.
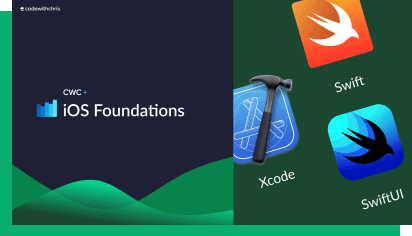
iOS Foundations (SwiftUI)
This course is designed for absolute beginners and will help you gain the skills to confidently build apps that fetch data from feeds, local files and APIs.
Module 1: The War Card Game
Lesson 1: The Apple Developer Ecosystem
- Apple Developer Ecosystem (1:19)
- Xcode (1:24)
- Swift (1:49)
- SwiftUI (1:59)
- Apple Developer Program (2:34)
- Provisioning Portal (2:55)
- Testflight (3:15)
- App Store Connect (3:30)
Lesson 2: Introduction to Xcode
- Where to get Xcode (0:54)
- Older Xcode versions (3:03)
- Starting a new project (4:00)
- File Navigator (7:04)
- Xcode Editor Area (7:35)
- Inspector Area (8:05)
- Xcode Toolbar (9:24)
- iOS Simulator (9:53)
- Project files (11:37)
- Navigating in Xcode (14:34)
Lesson 3: How to Build User Interfaces
- New Xcode project (1:18)
- iOS Simulator (1:43)
- Preview Canvas (2:43)
- Building the UI (4:38)
- SwiftUI Text View (6:08)
- Padding Modifier (6:34)
- Background Color Modifier (7:19)
- Building UI Visually (8:08)
Lesson 4: SwiftUI Views and Containers
- SwiftUI Image View (0:58)
- Adding Image Assets (1:47)
- Displaying Images (3:07)
- Resizable Modifier (3:27)
- Aspect Ratio Modifier (3:46)
- VStack element (4:58)
- HStack element (6:26)
- ZStack element (6:37)
- Spacer element (9:27)
Lesson 5: Build The War Card Game UI
- New Xcode project (0:41)
- Add Image Assets (1:12)
- Preview of the UI (2:39)
- Xcode Safe Area (3:15)
- Building the UI (6:30)
Lesson 6: Swift Variables and Constants
- View Lifecycle (1:03)
- Xcode Playgrounds (3:00)
- What is data? (4:43)
- Swift String (4:54)
- Comments (5:05)
- Swift Int (5:32)
- Swift Double (5:43)
- Swift Bool (5:55)
- Variables (6:38)
- Print function (8:17)
- Constants (12:29)
- Swift Functions (2:25)
- Function Parameters (4:07)
- Function Return Values (6:59)
- Multiple Function Parameters (11:53)
- Default Parameter Values (13:30)
- Function Argument Labels (15:02)
- Hiding Argument Labels (17:45)
- Function Signatures (19:02)
- Swift Structures (0:59)
- Declaring Structs (2:25)
- Struct Example (4:58)
- Variable Scope (7:56)
- Computed Properties (10:55)
- Creating Structure Instances (0:57)
- Structs as Data Types (4:05)
- Access Control and Access Labels (11:52)
- Example of Instances (14:17)
- SwiftUI Button Syntax (0:49)
- Trailing Closure (5:56)
- Button with an Image (8:18)
- SFSymbols (10:39)
- SwiftUI Button in the War Card Game (12:20)
- Creating properties for data (1:00)
- Referencing properties in view code (2:16)
- Implementing SwiftUI Button actions (5:09)
- State Property Wrapper (6:22)
- Randomizing Cards (9:04)
- Private Access Level (12:52)
Lesson 12: Swift If Statements
- Swift If Statements Syntax (0:46)
- Using “And” in conditions (2:27)
- Using “Or” in conditions (3:09)
- Combining “And” and “Or” (4:08)
- Swift Else if (5:22)
- Swift Else (6:42)
- If statement with other data types (7:56)
- Adding If conditions to the War Card Game (11:45)
Module 2: The Recipe List App
- Overview (0:00)
- Declaring Arrays (1:07)
- Appending Array Items (2:39)
- How Arrays Work (3:57)
- Accessing and Updating Arrays (5:21)
- Removing Array Items (6:43)
- Handy Array Methods (8:50)
- SwiftUI List Syntax (1:23)
- Dynamic List (4:00)
- NavigationView and NavigationLink (9:07)
- NavigationBarTitle (9:50)
- For-In Loop Syntax (0:52)
- For Loop with Arrays (4:15)
- Repeat While Loop (7:15)
- While Loop (9:26)
- Declaring Classes (0:48)
- Class Instances (1:44)
- Subclassing (3:27)
- Inheritance (5:35)
- Superclass/Subclass (6:18)
- Overriding Methods (8:13)
- Subclass vs Protocol syntax (10:38)
Lesson 6: Value vs Reference Types
- Value types and Reference Types (0:23)
- Structs vs Classes(1:11)
- Characteristics of Structs (1:43)
- Characteristics of Classes (5:52)
- When to use Classes vs Structs (8:27)
Lesson 7: MVVM Architecture Pattern
- Overview of MVVM (0:43)
- Using Groups to Organize Xcode Project (2:00)
- MVVM Example Implementation (4:38)
- ObservableObject Protocol (2:49)
- ObservedObject Property Wrapper (3:05)
- @Published Property Wrapper (4:05)
- Implicitly Unwrapped Optional (2:10)
- Optionals (5:20)
- Unwrapping Optionals (6:57)
- Optional Binding (10:44)
- Optional Chaining (12:54)
- When to use Optionals (16:51)
- How a Dictionary Works (0:39)
- Declaring an Empty Dictionary (1:35)
- Inserting into a Dictionary (3:01)
- Retrieving Dictionary Values (4:00)
- Update Dictionary Values (5:40)
- Removing Dictionary Values (6:00)
- Declaring Initialized Dictionary (8:00)
- Looping Through a Dictionary (9:25)
Lesson 11: Intro to JSON Data Format
- Reading JSON (1:19)
- JSON Objects (1:49)
- JSON Arrays (2:33)
- JSON Objects in Array (2:58)
- Creating a JSON file (3:15)
- Validating JSON (6:00)
- JSON File Example (10:08)
- Path to local JSON file (2:10)
- Create a Data object from JSON (6:20)
- Swift Error Handling (8:06)
- Parse the JSON (10:28)
- Mapping the Model to the JSON Object (11:48)
- Decodable Protocol (14:10)
- Setting Unique IDs for Model Objects (21:37)
- Adding Image Assets (2:16)
- Adding JSON file (2:33)
- Parsing JSON (5:22)
- Xcode Debugging (24:25)
Lesson 14: ForEach and ScrollViews
- SwiftUI ForEach (1:49)
- SwiftUI ScrollView (5:09)
Lesson 15: The RecipeDetail View
- Creating new SwiftUI Views (0:22)
- SwiftUI NavigationView (18:08)
- NavigationBarTitle (18:20)
- SwiftUI NavigationLink (18:40)
Module 3: Git and GitHub
Lesson 1: Introduction to Git and GitHub
- What is Git? (1:04)
- What is GitHub? (1:20)
- How Git works (1:35)
- Commits (1:40)
- Branching (2:07)
- Local and Remote Repos (2:31)
- Staging and Commit (3:24)
- Putting it all together (4:41)
- Branching (5:45)
- Cloning (6:14)
- Forking (6:53)
- Ways to use GitHub (7:35)
- GitHub Signup (0:10)
- Create a repository (2:16)
- Create an Xcode project (4:23)
- Add GitHub account to Xcode with Personal Access Token (5:25)
- Commit from Xcode (10:40)
- Push to GitHub (12:30)
- Connecting to GitHub from Xcode (12:45)
- Adding new files (0:00)
- Commit new files (0:35)
- Push changes (4:59)
- Fetch changes (7:32)
- Clone repo on different machine (8:24)
Module 4: Recipe App
- Overview (0:00)
Lesson 2: Adding the Project to GitHub
- Creating a copy of the project (0:32)
- Adding source control to existing project (1:25)
- Creating repo on GitHub (2:25)
- Adding remote repo to Xcode (3:43)
- Pushing to remote (3:50)
- SwiftUI TabView (0:30)
- Customizing tabs (1:18)
- Detect tab selection (4:01)
- Two way bindings (5:40)
- Tab tags (7:30)
- Adding tabs to recipe app (9:00)
- Rectangle (0:33)
- Frame (1:06)
- Alignment (1:44)
- GeometryReader (4:18)
- Relative sizes (5:45)
- onTapGesture (7:00)
- GeometryReader frame (7:28)
- Offset modifier (18:24)
- Padding vs Offset (18:48)
- Position modifier (21:16)
- Adding new JSON (2:08)
- Fixing JSON parsing (2:52)
- Browsing GitHub commits (10:02)
- Adding new SwiftUI View (0:59)
- Review data flow options (3:58)
- environmentObject modifier (6:38)
- Implementing environmentObject in Xcode (7:20)
- Swipe-able cards using tabViewStyle and PageTabViewStyle (5:34)
- Drop Shadow modifier (8:43)
- Customize the shadow effect (17:19)
- Hiding NavigationBarTitle (2:12)
- List with ScrollView and ForEach (4:09)
- LazyVStack (4:50)
- Picker basics (0:40)
- Picker selection (2:15)
- Picker options (3:00)
- Picker tag (5:28)
- Picker style (7:33)
- MenuPickerStyle (7:53)
- SegmentedPickerStyle (8:59)
Lesson 10: Calculating Ingredient Portions
- Calculating portions math walkthrough (0:00)
Lesson 11: Recipe Serving Size Picker
- Checking documentation (0:49)
- Implementing Picker (1:39)
- Comparing source control version (4:47)
- Discard changes (6:20)
- Portion calculation pseudo-code (12:50)
Lesson 12: Displaying Ingredient Portions
- Calculate portions (0:00)
- Nil coalescing operator (0:50)
- Modulo operator (6:30)
- suffix method (18:10)
Lesson 13: Featured View Details
- Recipe detail in popover view using .sheet modifier (2:00)
- Recipe prep time (12:25)
- array firstIndex method (15:40)
- onAppear modifier (18:30)
- Recipe highlights (20:10)
- SwiftUI custom fonts (0:35)
- SwiftUI system fonts (3:07)
- font modifier with custom font (3:40)
Module 5: Learning App
- Overview (0:00)
- What you’ll learn (0:59)
- User navigation architecture (0:20)
- View hierarchy and data flow architecture (2:29)
- Creating the Xcode project (4:16)
- Renaming basic files (5:09)
- Creating folders and organizing project (6:13)
- Creating the ViewModel (7:10)
- Committing to local repo (9:08)
- Creating and connecting remote GitHub repo (9:57)
- Pushing to remote repo (10:53)
Lesson 3: Parsing the JSON Data
- Adding assets and resources (0:17)
- Structure of the JSON data file (1:48)
- Going through the JSON file (3:04)
- Overview of displaying text with HTML and CSS (4:47)
- Going through the ‘test’ object of the JSON (7:02)
- Creating models for the JSON data (8:41)
- Parsing the JSON data in ViewModel (13:53)
- Check the parsing with breakpoints (19:05)
- Parsing the style data (21:10)
- Committing and pushing to repo (23:38)
Lesson 4: Building the HomeView
- Breaking down the design (0:13)
- Layout of a card and navigation (1:13)
- Creating the HomeView card list (3:40)
- Fixing the Preview with the environment object (4:46)
- Styling the card rectangle (5:32)
- Styling the card image (8:00)
- Styling the card text and icons (8:46)
- Fixing the spacing (11:20)
- Implementing dynamic values for cards (13:48)
- Implementing a reusable card subview (16:13)
- Adding the HomeView title and NavigationView (20:46)
Lesson 5: Building the Lesson List View
- Overview of lesson list layout and app flow (0:15)
- First approach for the lesson list ContentView (2:01)
- Tracking the current module and indices (5:53)
- Improved ContentView using current module (11:02)
- Creating and styling lesson cards (12:12)
- Implementing dynamic values for ContentView (14:55)
- Fixing styling and spacing (17:30)
- Extracting lesson cards into a subview (21:00)
- Overview of app flow and design (0:15)
- Creating ContentDetailView (1:08)
- Navigating to the ContentDetailView (2:53)
- Adding the video player (7:34)
- Video URL hosting (8:52)
- Creating Constants file for URLs (11:50)
- Calling the video URL (12:30)
- Adding the next lesson button (16:06)
- Implementing the button action (18:37)
- Adding dynamic button text (21:41)
- Creating a test project using UIViewRepresentable (1:06)
- Implementing makeUIView (2:11)
- Creating a demo mapView (3:29)
- Implementing updateUIView (4:22)
- Updating ContentView and demoing (5:32)
- Implementing a UITextView (6:17)
- Overview of WebView attributed string alternative (7:23)
- Implementing the lesson description using UITextView (8:53)
- Getting each description from the JSON (11:18)
- Turning HTML string to NSAttributedString (14:08)
- Two techniques of error handling with NSAttributedStrings (20:30)
- Setting lesson description for each lesson (23:20)
- Scrolling text back to top (25:10)
- Adding lesson title (26:42)
Lesson 8: A Closer Look at NavigationLink
- Setting up demo project (0:21)
- Adding NavigationLink (1:52)
- Tracking selected navigation (3:40)
- Modifying and unwinding the selection (8:26)
- Adding selection tracking to Learning App (12:02)
- Adding ‘Complete’ button to last lesson (15:51)
- Creating the reusable rectangle card (18:30)
- Commit and push to repo (20:53)
- Overview of quiz design (0:12)
- Creating TestView (0:45)
- Adding navigation to TestView (2:03)
- Adding TestView content (4:34)
- Reviewing beginModule and beginLesson (7:50)
- Creating beginTest to set current question (9:57)
- Displaying question number (13:17)
- Displaying question content (15:12)
- Refactor CodeTextView (17:55)
- Checking modifications (19:00)
- Committing and pushing to repo (19:31)
- Editing spacing of TestView (0:18)
- Implementing quiz answer ScrollView (1:12)
- Adding styling and buttons (3:51)
- Implementing selection action (5:54)
- Making the entire button tappable (8:25)
- Adding the submit button (9:22)
- Disabling submit button for no selection (14:36)
- Displaying submission correctness (16:27)
- Committing and pushing to repo (23:24)
Lesson 11: Quiz Question Navigation
- Overview of quiz question navigation (0:12)
- Implementing nextQuestion function (2:04)
- Calling nextQuestion navigation (5:44)
- Changing submit button text after press (8:49)
- Committing and pushing to repo (13:25)
- Overview of quiz result interface (0:12)
- Creating quiz result view (0:41)
- Adding complete button (2:51)
- Incorporating the result view (4:49)
- Showing number of correct answers (7:15)
- Making result title dynamic (9:16)
- Debugging and calling the dynamic title (13:06)
- Guarding for errors (14:31)
- Committing and pushing to repo (17:31)
- Overview of the second JSON module (0:12)
- Hosting the JSON (1:38)
- Setting up GitHub Pages hosting (2:07)
- Navigating to the GitHub page URL and 404 errors (4:52)
- Next lesson overview (6:53)
- Advantage of hosting JSON remotely (7:24)
- Overview of accessing remote data (0:14)
- Getting remote data from URL (1:09)
- Using URLSession (4:53)
- Kicking off the data task (7:41)
- Handling and decoding the JSON data (9:34)
- Checking the request (14:58)
- Final words and next steps (16:03)
Lesson 14 Follow Up: Updating View Code from a Background Thread
- Warnings about updating views through background threads (0:00)
- Making changes through background threads with DispatchQueue (1:46)
Module 6: City Sights App
- Overview (0:00)
- What you’ll learn (0:29)
Lesson 2: App Architecture and Set Up
- User navigation (0:18)
- View hierarchy and data flow (2:33)
- Creating repo on GitHub (4:30)
- Creating Xcode project (5:00)
- Connecting GitHub repo to Xcode project (6:05)
- Creating and renaming files (6:25)
- Committing and pushing to repo (8:06)
- Cleaning view names (0:16)
- Reviewing the view hierarchy (1:15)
- Implementing ContentModel (2:08)
- Requesting location permission (4:27)
- Overview of geolocating the user (5:40)
- Editing Info.plist to enable location permission requests (6:28)
- Testing the location permission popup (8:45)
- Simulator location popup settings (9:48)
- Detecting user permissions (10:37)
- Conforming to CLLocationManagerDelegate (11:57)
- Implementing location manager delegate methods (15:35)
- Testing location permissions (19:03)
- Getting the location of the user (20:55)
- Getting started with the Yelp API (0:20)
- Getting API authorization code (4:39)
- Capturing user location (7:24)
- Implementing Yelp API methods to get businesses (8:40)
- Creating URL (11:26)
- Using URLComponents (14:07)
- Creating URL request (18:36)
- Implementing URLSession and data task (22:31)
- Checking the request response (24:07)
- Tracking requests with Proxyman (25:44)
Lesson 4 Follow Up: API Key Security
- Keeping your API Key private (0:00)
Lesson 5: Parsing the Yelp API JSON
- Checking API response (0:19)
- Creating Business model (2:17)
- Creating BusinessSearch model (8:14)
- Parsing the JSON response (10:00)
- Testing the response (12:00)
- Assigning the results (13:09)
- Extracting strings to Constants file (15:55)
- Using switch statements (18:00)
- Final checks and pushing to repo (19:26)
Lesson 6: Building the Business List View
- Overview of screens/ views (0:12)
- Detecting authorization status (2:40)
- Implementing the HomeView (6:00)
- Adding ProgressView in HomeView (7:11)
- Creating list view (8:13)
- Testing the UI (12:20)
- Displaying the business list (13:12)
- Using Sections (16:38)
- Creating reusable section headers (19:50)
- Creating business section view (21:59)
Lesson 7: Building the Business Row
- Changing model property names with CodingKeys (0:19)
- Overview of business row design (5:02)
- Creating BusinessRow view (7:02)
- Displaying business ratings (10:07)
- Formatting distance text (13:36)
- Approach to displaying images (16:00)
- Getting image data (17:30)
- Displaying business image (21:54)
- Calling get business images function (24:40)
- Sorting businesses by distance (26:24)
- Resizing business images (28:45)
- General styling (29:28)
- Making each BusinessRow tappable (0:15)
- Adding NavigationView (3:50)
- Overview of BusinessDetail view (6:55)
- Implementation strategy (10:00)
- Introducing Links (11:04)
- Implementing BusinessDetail view (13:51)
- Committing and conclusion (36:45)
- Creating BusinessMap using MapKit (0:12)
- Display map in HomeView (4:50)
- Add annotations to map (6:50)
- Annotations demo (14:45)
- Adding dismantleUIView (17:08)
Lesson 10: Handling Events from UIKit Controls
- Overview of handling events (0:26)
- Explaining the delegate (1:20)
- Diagram of protocol delegate pattern (4:05)
- How UIViewRepresentable helps incorporate protocol delegate (5:38)
- Implementing coordinator (7:32)
- Making the delegate (14:38)
- Accounting for blue dot (16:50)
- Making annotation view reusable (18:12)
Lesson 11: Map View – Viewing the Business Detail
- Overview of showing business details (0:13)
- Add sheet modifier to BusinessMap (1:45)
- Passing Binding to BusinessMap (3:56)
- Implementing tapped mapView method (6:50)
- Getting model data in mapView (9:00)
- Continue implementing tapped method (11:05)
- Adding top-bar to map (14:35)
- Overview of design(0:10)
- Implementing DirectionsView (1:15)
- Creating BusinessTitle (2:44)
- Implementing get directions button (4:45)
- Fixing multiple views issue (7:52)
- Adding map link (8:40)
- Encoding string in URL (12:40)
- Creating DirectionsMap (14:50)
- Implementing starting points and endpoints in map (17:56)
- Calculating directions (25:08)
- Drawing the route line (27:18)
- Zooming into region (30:50)
- Add location showing (32:02)
- Update updateUIView and dismantleUIView (34:20)
Lesson 13: Onboarding Sequence
- Overview of onboarding design (0:10)
- Strategy for onboarding (1:40)
- Implementing OnboardingView (2:51)
- Adding location request function (9:00)
- Setting background colours and styling (12:38)
- Implementing LocationDeniedView (19:25)
- Adding settings URL (23:48)
- Overview (0:00)
- Adding Yelp badge (1:00)
- Adding dashed dividers using Path (6:48)
- Implementing dynamic city names with CLGeocoder (14:06)
Free Swift and SwiftUI Tutorials
Swift Tutorials
- Complete Swift for Beginners
- Swift Video Tutorial
- Swift Video Tutorial Resources
- Swift 3 Hour Masterclass
- Learn Swift
- Swift Cheat Sheet
- Swift Try Catch Error Handling
- Swift Enum
- Swift Random Numbers
- Swift Loops
- Swift Guard Statement
- Swift Strings
- Swift Arrays
- Swift Dictionaries
- Swift Variables
- Swift Data Types
- Swift If Statements
- Swift Loops Video Pt. 1
- Swift Loops Video Pt. 2
- Swift Switch Statement
- Swift Functions Pt. 1
- Swift Functions Pt. 2
- Swift Optionals
- Swift Math Operators
- Swift Classes
- Swift Structures
- Swift Initializers
- Swift Subclassing
- Designated and Convenience Initializers
- Swift Properties
- Swift Challenges (solutions)
UIKit
SwiftUI Tutorials
- SwiftUI Resource Hub
- What is SwiftUI?
- SwiftUI Tutorial
- SwiftUI Image
- SwiftUI TabView
- SwiftUI ScrollView
- SwiftUI Stacks
- SwiftUI Picker
- SwftUI Color
- SwiftUI TextField
- SwiftUI Button
- SwiftUI List
- Building UI with SwiftUI
- SwiftUI Basic Views and Containers
- Basic SwiftUI Layout and Elements
- SwiftUI Modifiers
- War Card Game UI in SwiftUI
- SwiftUI View Update Lifecycle
- SwiftUI Buttons
- SwiftUI Button 1 Min Video
- SwiftUI State Properties
- Another State Properties Video
- SFSymbols
- SwiftUI Tutorial: Build a Slots App
- SwiftUI Data Flow and View Composition
- Improving the Slots app
- SwiftUI Animations
- SwiftUI Basics Tutorial
- SwiftUI NavigationView
- SwiftUI Handling User Input
- SwiftUI War Card Game
- SwiftUI vs UIKit
- Creating Circular Images
- How to Align 3 Images
- SwiftUI Displaying a Map (MapKit)
- SwiftUI CoreLocation
- SwiftUI Map Annotations
- SwiftUI Launch Google Maps
- SwiftUI Launch Apple Maps
- SwiftUI Custom Fonts
App Development
Xcode Tutorials
- Xcode 12 Walkthrough
- Xcode Tutorial for Beginners
- Xcode Video Tutorial
- Save Xcode Hard Drive Space
- Xcode No Storyboard Fix
- Where’s the Assistant Editor?
- Where’s the Library Pane?
- Xcode 10.2 on High Sierra
- Reseting Xcode Simulator
- What’s new in Xcode 11
- Xcode Debugging Demo
- Xcode Shortcuts
- Xcode Tip: Changing Simulator Size
- UI Testing in Xcode
Misc
8 Comments
Hello Chris,
Your teaching method is extremely effective. Keep up the great work. By the way, which recording software do you use to create the video tutorials.
Lots of luck in your future endeavors.
KC
Hello KC, thanks for the encouragement! Currently I’m using Camtasia to do screen recording.
How so you make text
Scroll across the screen using the new sprite kit?
Hey Randall,
I plan to cover SpriteKit in the future but for now, Ray’s got a great Sprite kit tutorial: http://www.raywenderlich.com/42699/spritekit-tutorial-for-beginners
Hope that helps!
Hey Chris, I was wondering if you are going to be talking about rss feeds in the next tutorial series? When are you expecting to have the nest tutorials out? Thanks
Hey Jon,
Working with RSS, Json and other types are feeds/data is going to be an upcoming topic. I’ve been releasing videos but forgot to update this page. Please check either Facebook or YouTube for the latest until I get a chance to update this page!
As for when the videos for working with RSS feeds will be released, i can’t give you an exact date, but it will probably be end of this month.
Thanks!
Hey, trying to work on a new app, but can’t seem to get the Store Kit working. Any vids on that subject? from start to finish?
Thanks Chris.
Hey Mike, great web app, godothings!
Unfortunately not at the immediate time but probably in December.
Have you tried looking at Ray Wenderlich’s stuff? I think he has some iOS7 books that might cover Store Kit.
Thanks for stopping by!